TIPS AND BEST PRACTICES
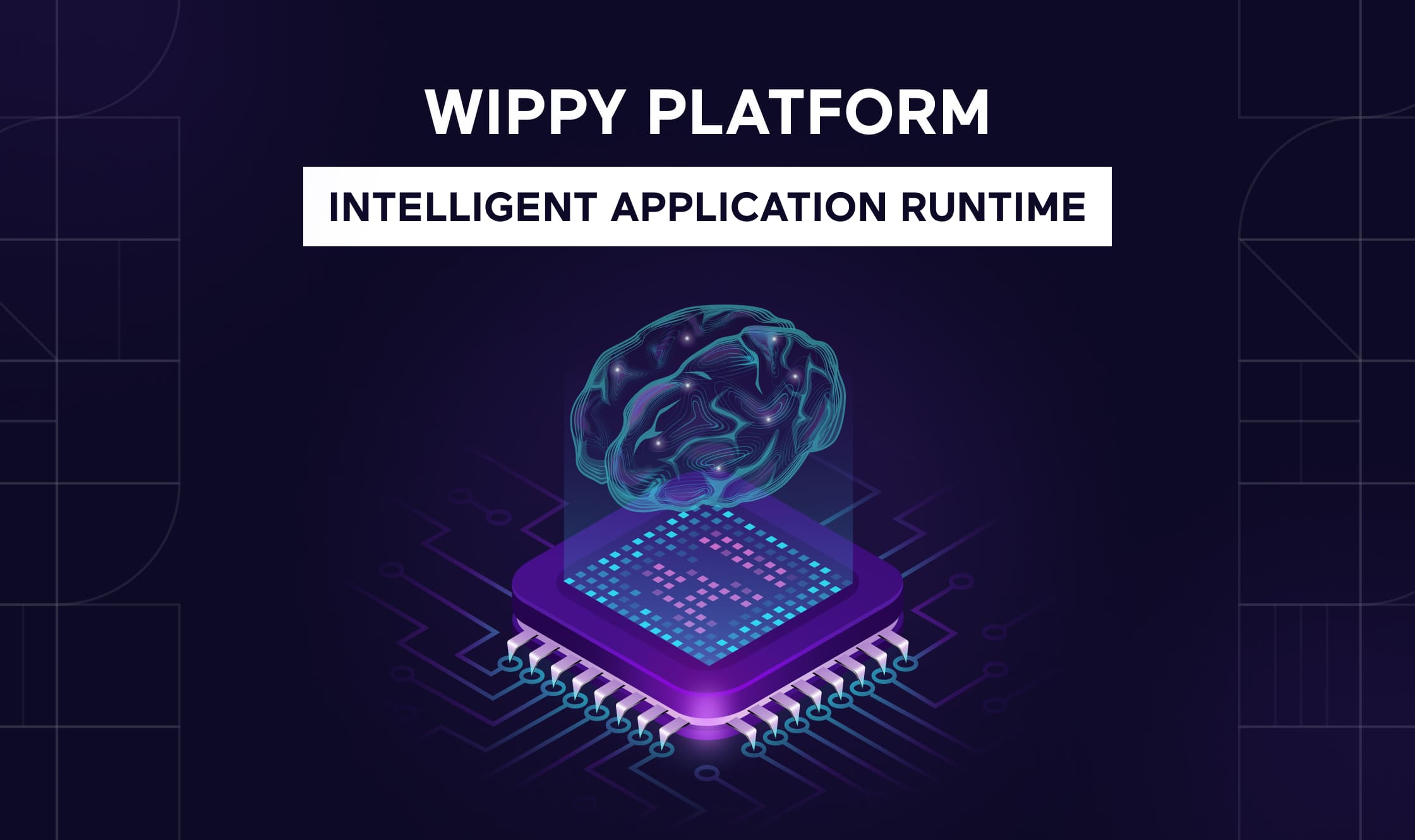
Wippy Platform: The Intelligent Application Runtime
In today’s rapidly evolving digital landscape, businesses need platforms that are not only robust and scalable but also intelligent and adaptable. The Wippy Platform, built upon the high-performance Wippy Runtime, is a groundbreaking system designed to empower developers and organizations to build, deploy, and manage sophisticated, AI-integrated applications with unprecedented speed and flexibility. It moves […]
Trending
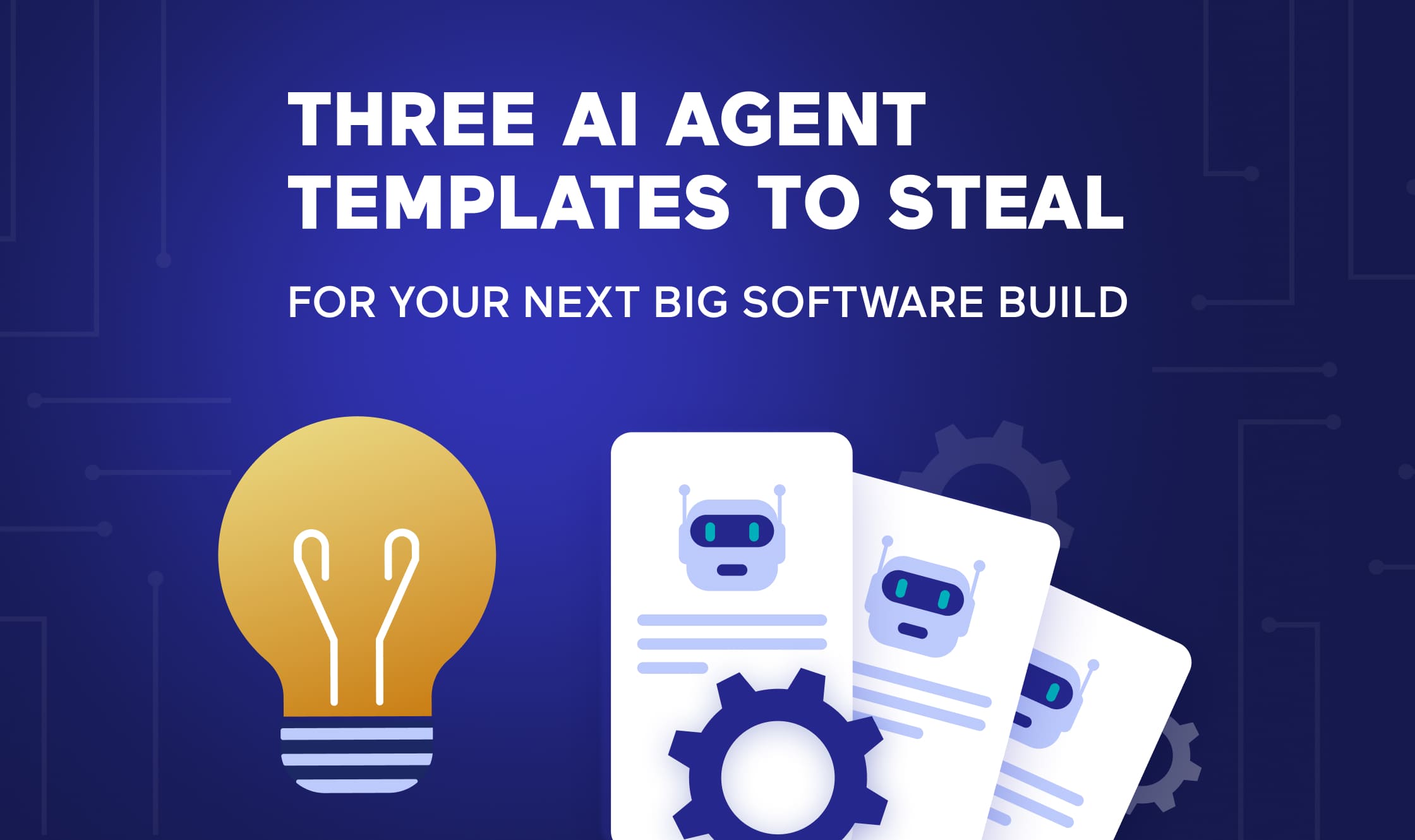
3 AI Agent Templates to Steal for Your Next Big Software Build
These aren’t ideas. They’re working products powered by Wippy. Most companies talk about “AI integration.” We talk about AI agents doing real work. While others are still testing copilots and chatbots, we’ve seen companies radically improve software development workflows with autonomous, self-modifying AI agents running in production. Not prototypes – actual shipped tools solving painful […]
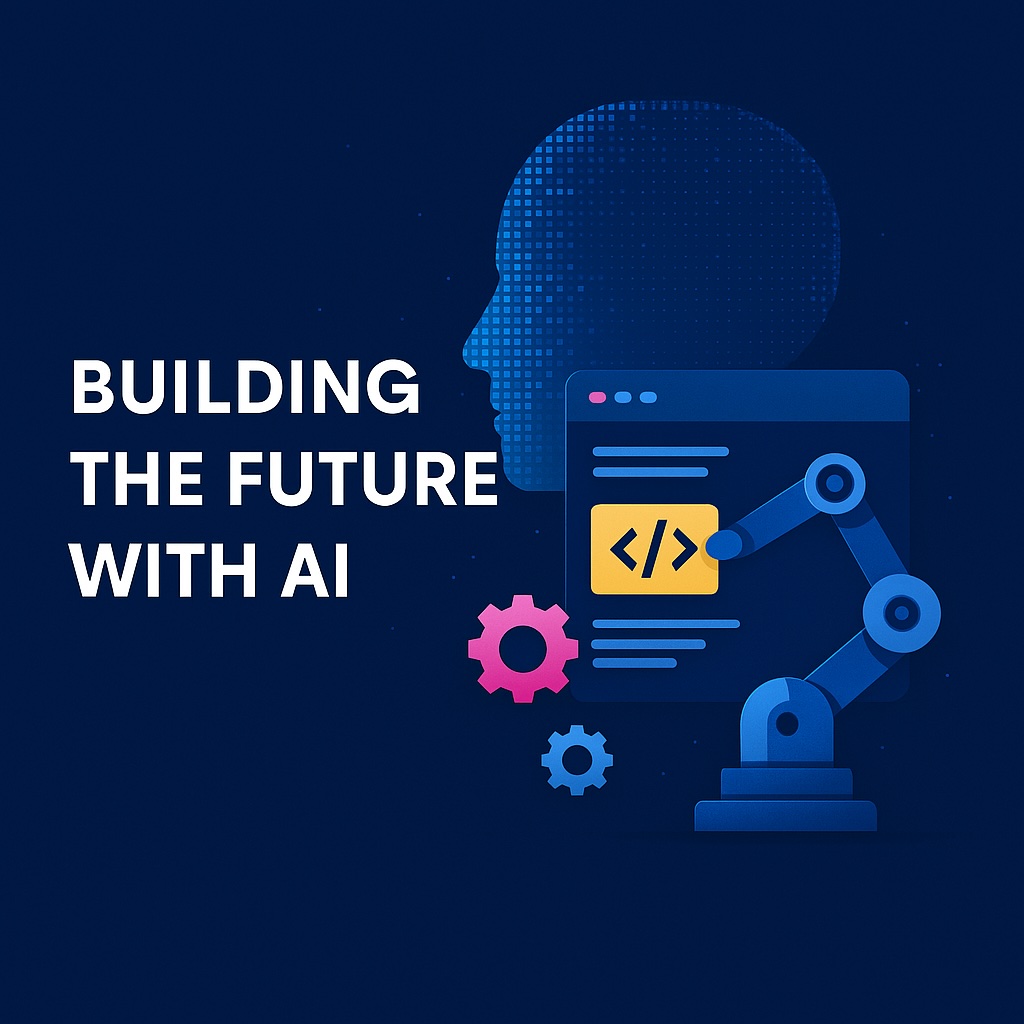
Transforming Backend Development with Self-Build Software for AI
Spiral Scout is leading a new era with self-build software backends. This innovative approach leverages AI-powered solutions to enhance developer workflow automation and AI integration, empowering teams to create adaptable, efficient systems tailored to their needs. In today’s tech landscape, integrating AI into software creation is essential. At Spiral Scout, we’re transforming Wippy from a […]
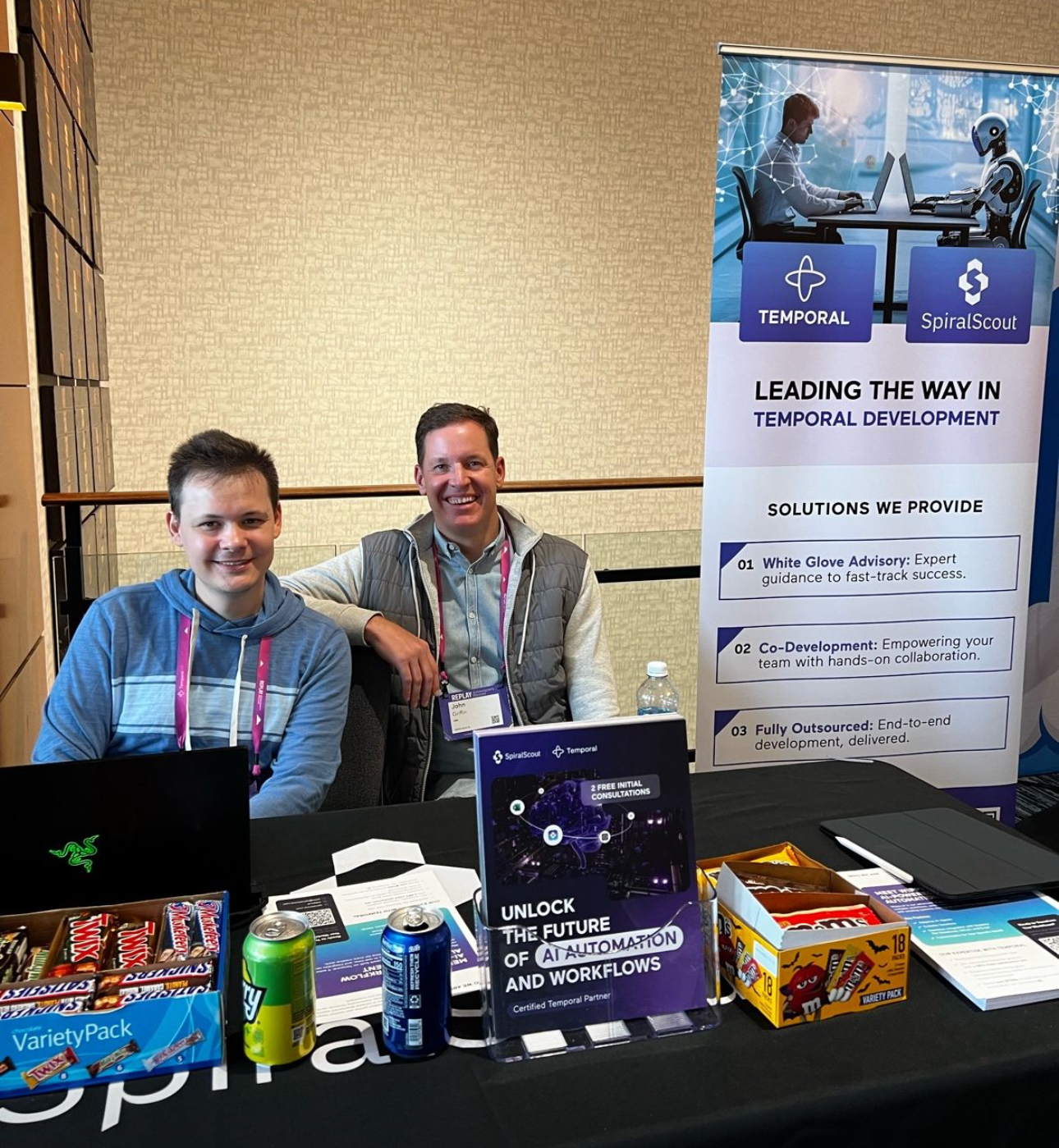
How AI is Transforming My Life and Work
The integration of AI into my daily routine has been transformative. As the co-founder and investor in multiple businesses, it’s not just about saving time; it’s about augmenting my capabilities, solving problems faster, and finding creative solutions I wouldn’t have considered otherwise. Here’s how I’ve been leveraging AI solutions for businesses to maximize efficiency and […]
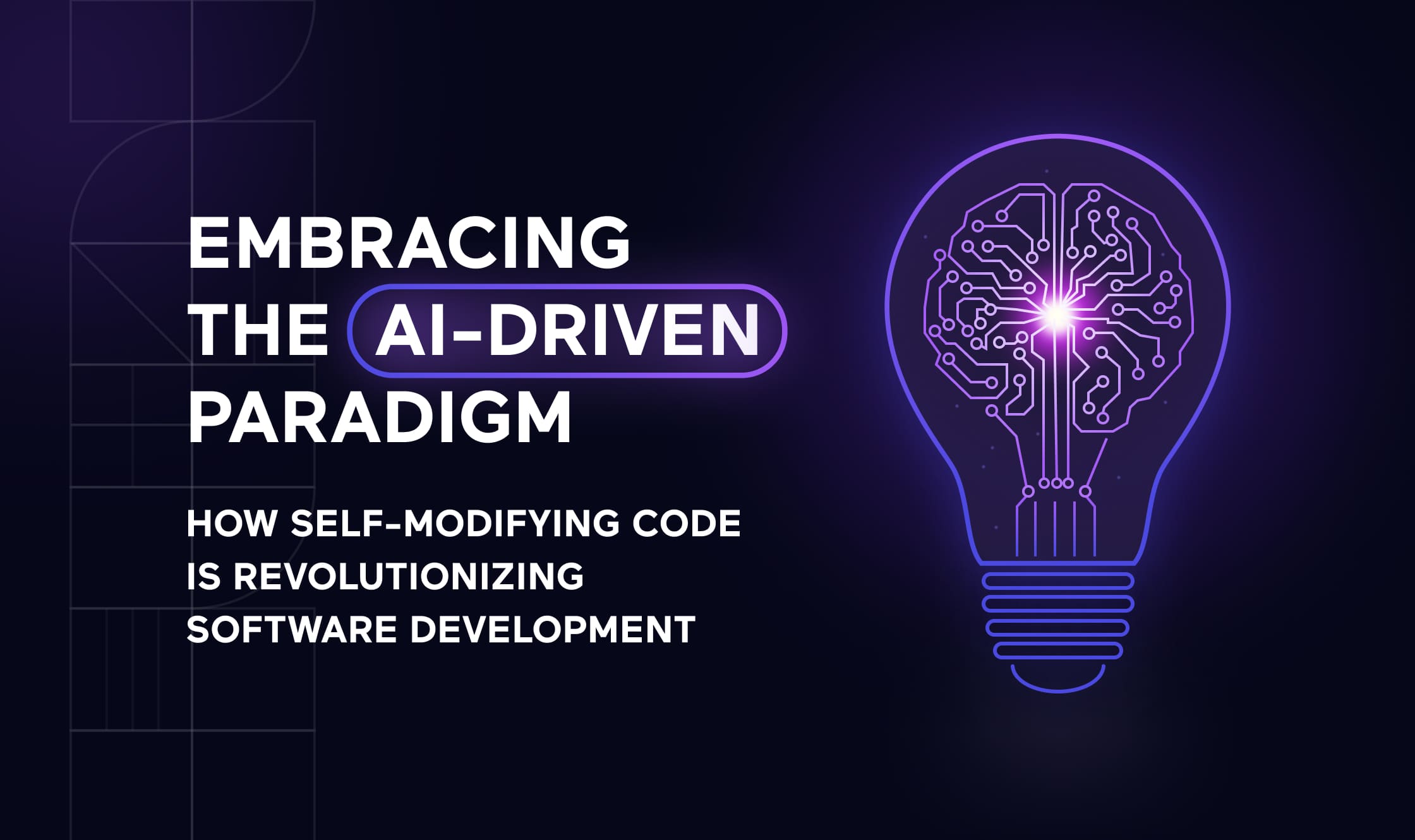
Self-Modifying AI Code: Redefining Software Development Paradigms
Self-modifying AI code is reshaping the software development landscape. By leveraging AI agent automation, developers can create systems that understand and improve themselves, reducing manual effort and enhancing adaptability. In the rapidly evolving software development landscape, a new paradigm is emerging—one that leverages artificial intelligence (AI) to fundamentally change how we write, maintain, and interact […]
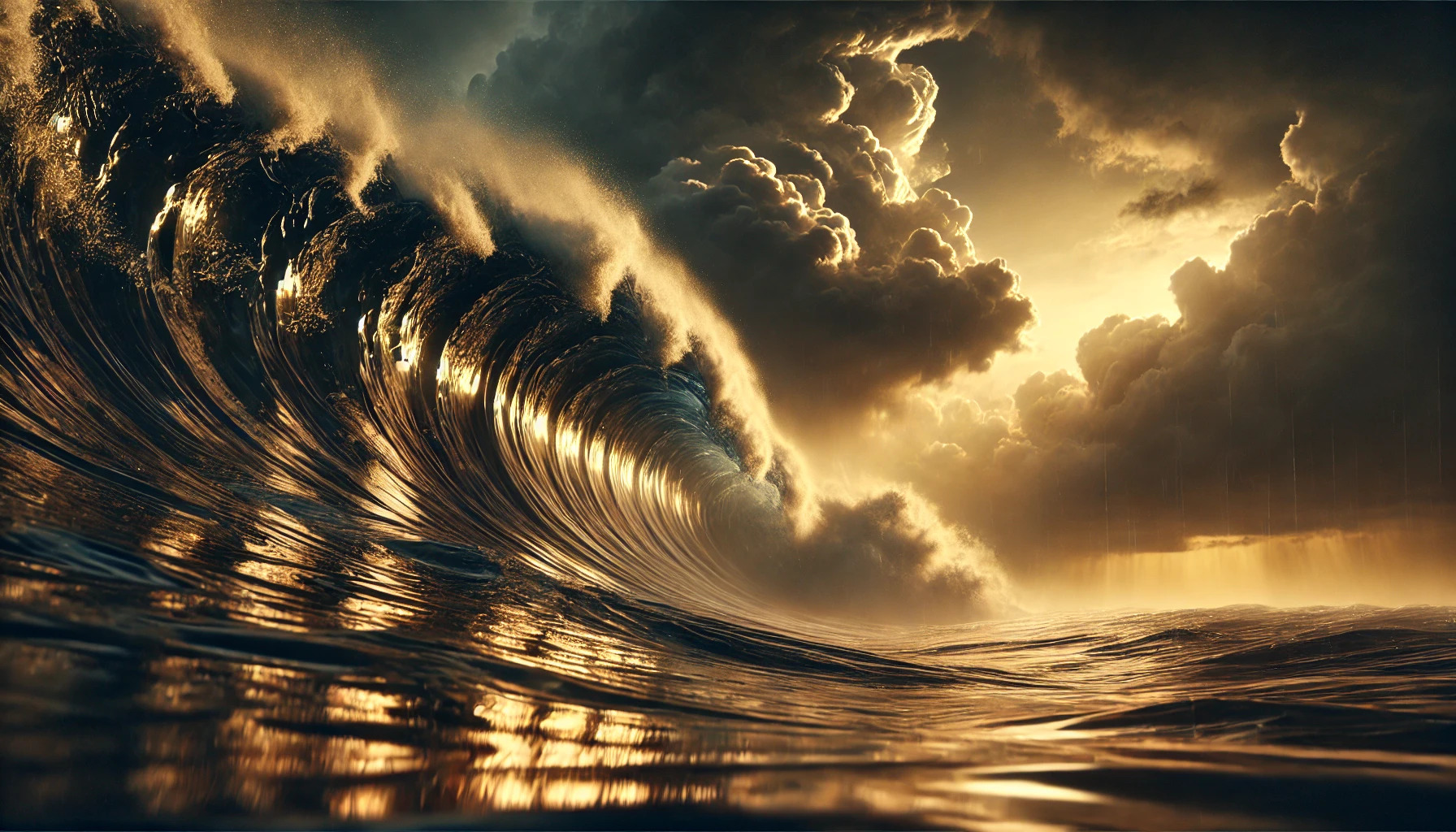
Wippy: Leading the Future of AI-Powered Business Automation
The way businesses approach automation is changing fast. SaaS transformed software over the last 25 years, but now we’re seeing a shift toward AI-powered Development platforms that leverage digital employees or agents that blend software and services in ways SaaS never could. The rise of AI Agents Platforms or Agents as a Service Platforms shows […]
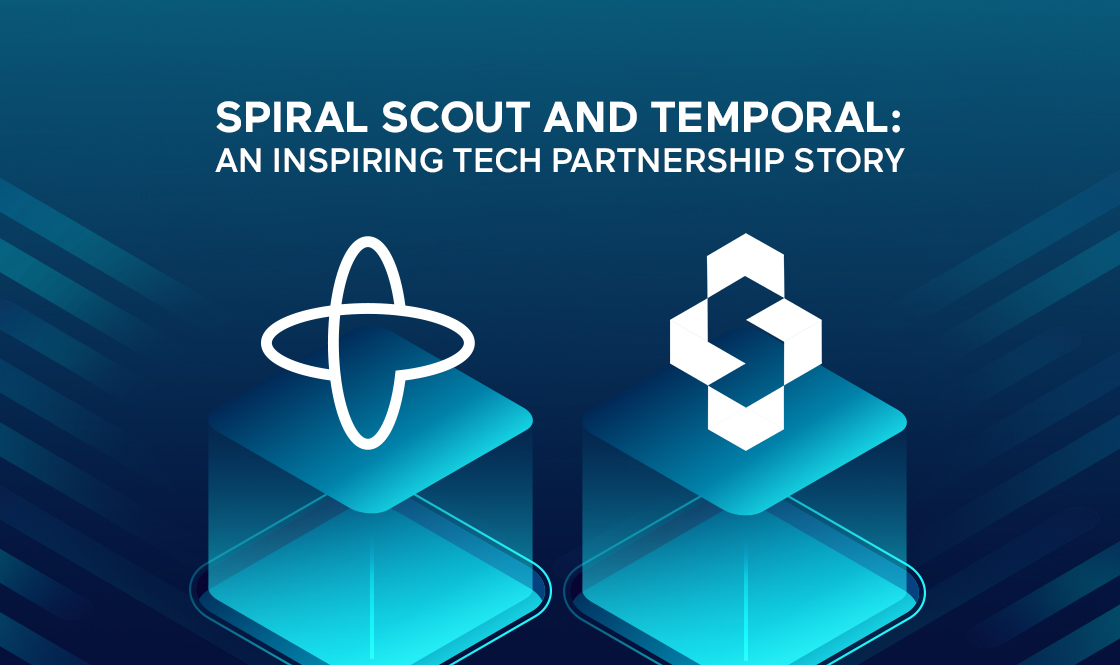
Insights into Spiral Scout’s Strategic Partnership with Temporal
Spiral Scout’s strategic partnership with Temporal represents a significant advancement in workflow automation solutions. By integrating Temporal’s technology, Spiral Scout provides comprehensive Temporal integration services, enhancing efficiency and innovation in software development. Back in 2019, JD, Spiral Scout’s co-founder and CTO, embarked on building a workflow engine. During his research, he discovered Temporal.io, a solution […]
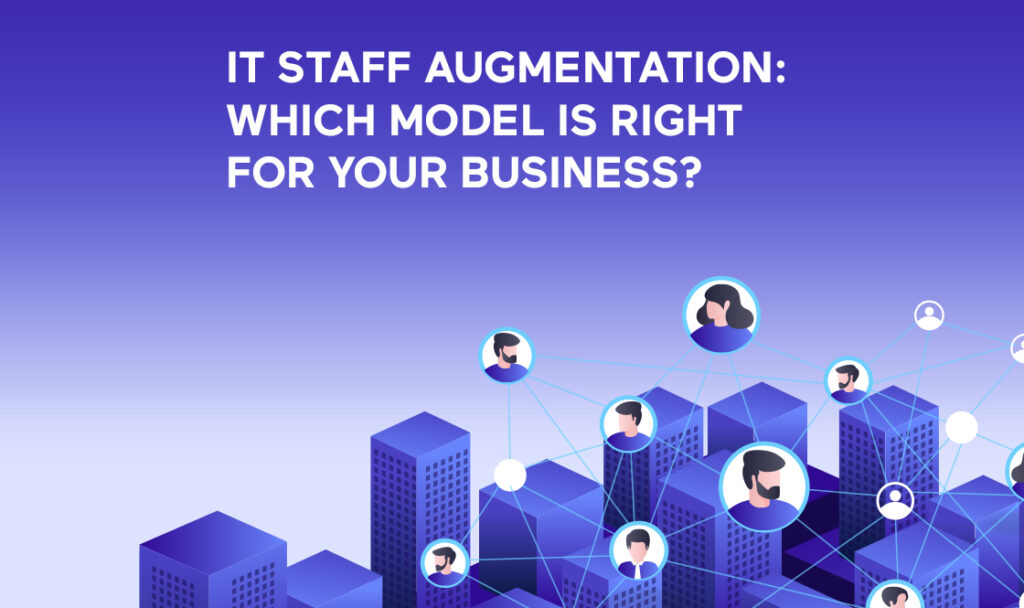
IT Staff Augmentation: Which Model is Right For Your Business?
In today’s fast-paced business environment, IT staff augmentation provides a flexible solution for accessing the right talent at the right time. This approach allows businesses to implement technologies quickly and effectively, staying competitive without the long-term financial implications of expanding the payroll. As companies strive to stay competitive, the need for skilled IT professionals has […]
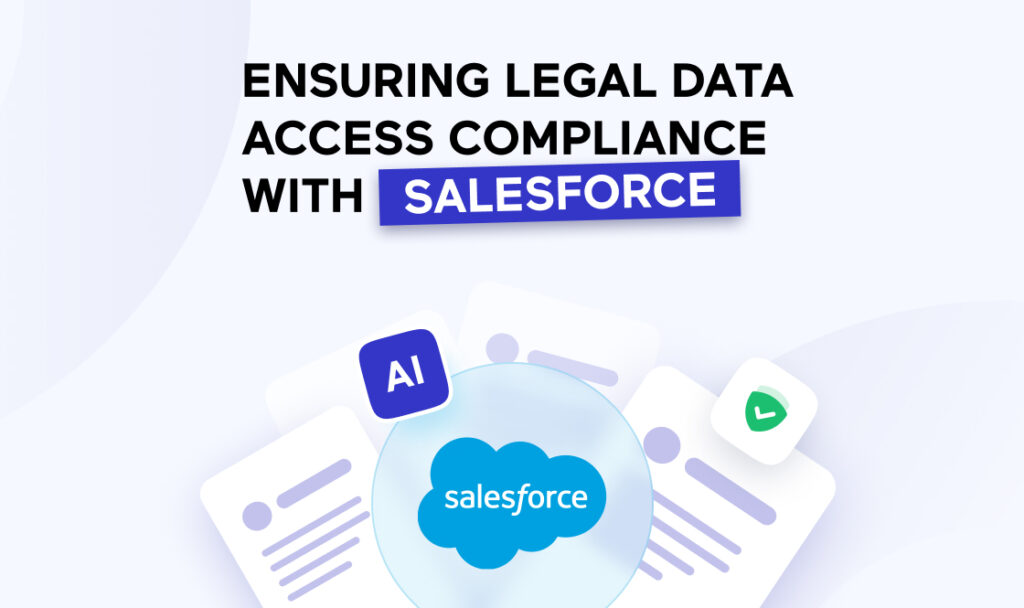
Master Legal Data Access Compliance in Salesforce
Dealing with complex data access laws is a significant challenge for legal teams. With Salesforce data compliance, businesses can navigate these challenges efficiently, minimizing the risk of costly errors and enhancing legal operations. Navigating the intricate landscape of data access and privacy laws is one of the most taxing responsibilities for legal teams today. With […]
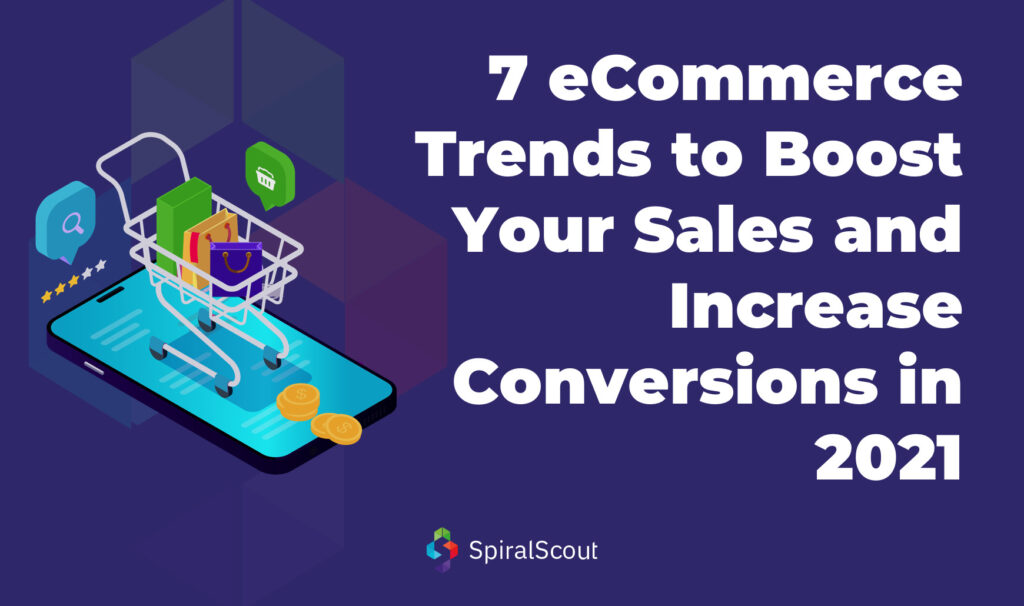
7 eCommerce Trends To Boost Your Sales and Increase Conversions
In the ever-evolving digital world, staying ahead of eCommerce trends is critical for businesses to thrive. From adopting headless architecture to AI-driven personalization, this article outlines seven impactful strategies that can help you implement effective boost online sales strategies, improve eCommerce conversion optimization, and enhance user experience. Whether you’re starting a new store or upgrading […]
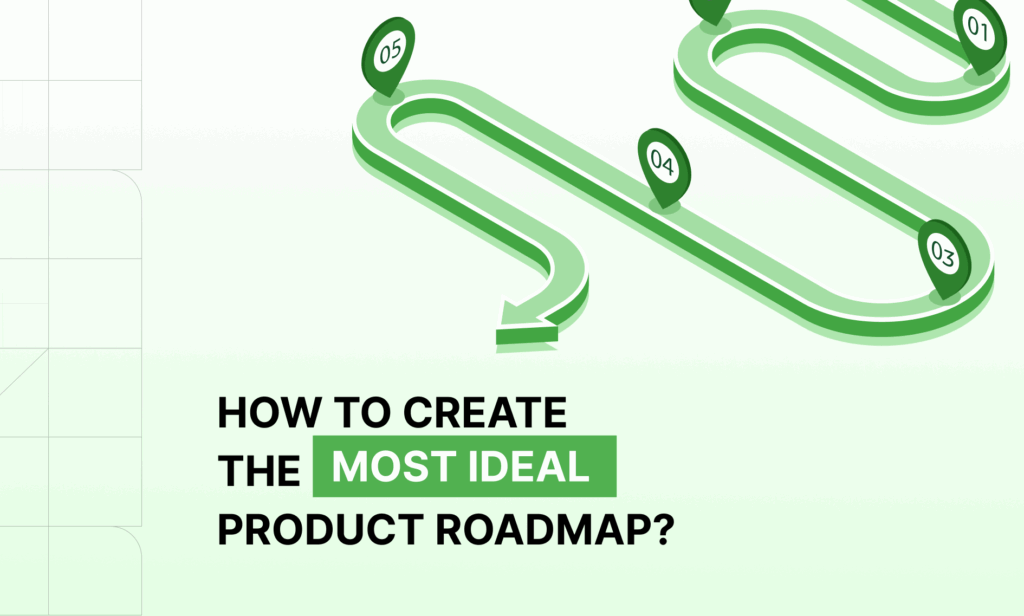
How to Create the Most Ideal Product Roadmap?
A well-crafted product roadmap is essential to align stakeholders, prioritize development efforts, and guide a product’s growth from ideation to launch. This guide walks you through the types, best practices, tools, and strategic insights—like integrating AI in product discovery and outsourcing—to help you build a roadmap that drives product success. Have questions? Let’s chat. What […]
Editor’s pick
AI Expertise
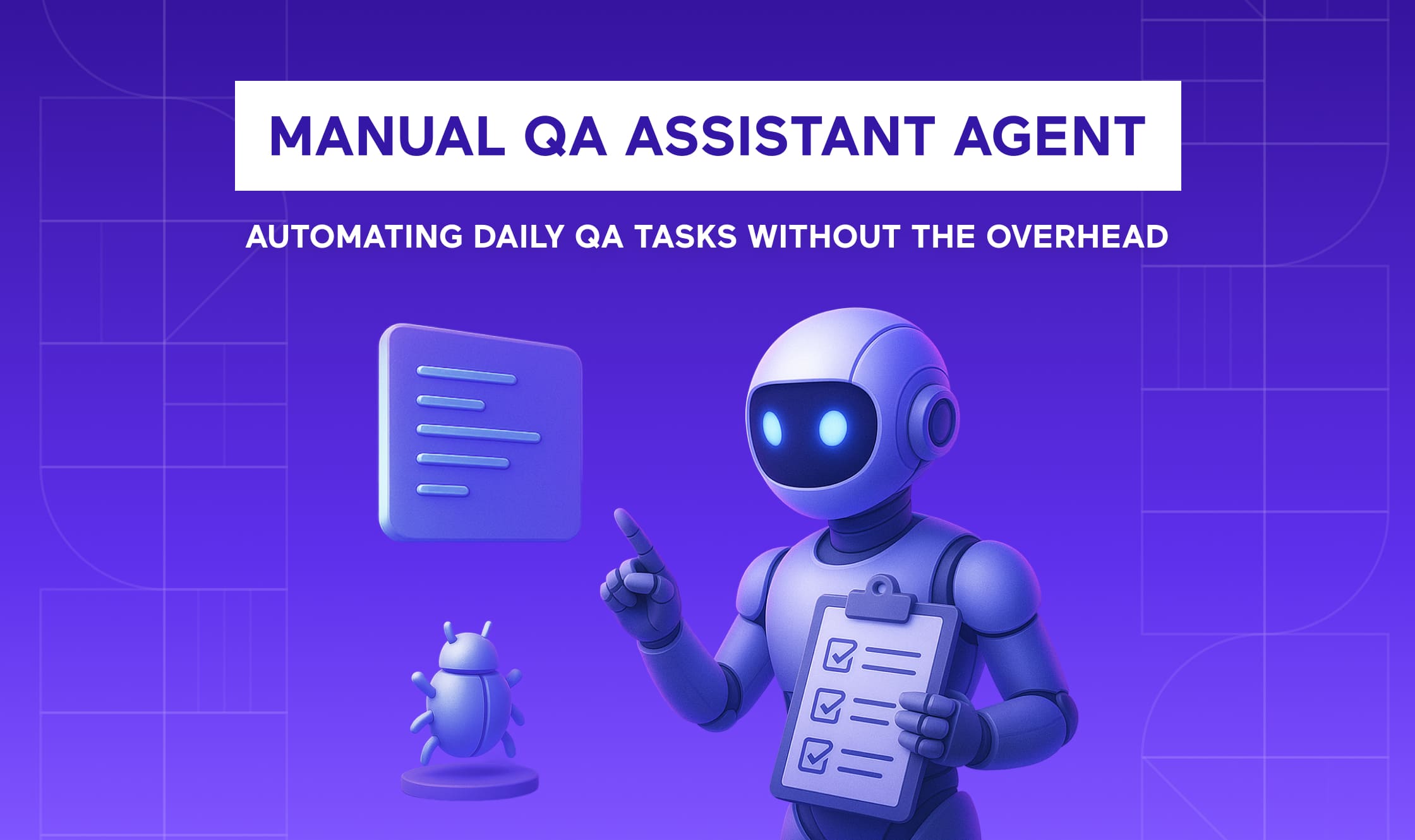
Meet the Manual QA Assistant Agent: Automating Daily QA Tasks Without the Overhead
Your manual QA team deserves better tools. Spiral Scout’s new AI-powered Manual QA Assistant Agent is built to help teams handle everyday grunt work – creating tickets, reading docs, extracting test data, scanning pages – so QAs can spend less time on busywork and more time ensuring product quality. What Does the Manual QA Assistant […]
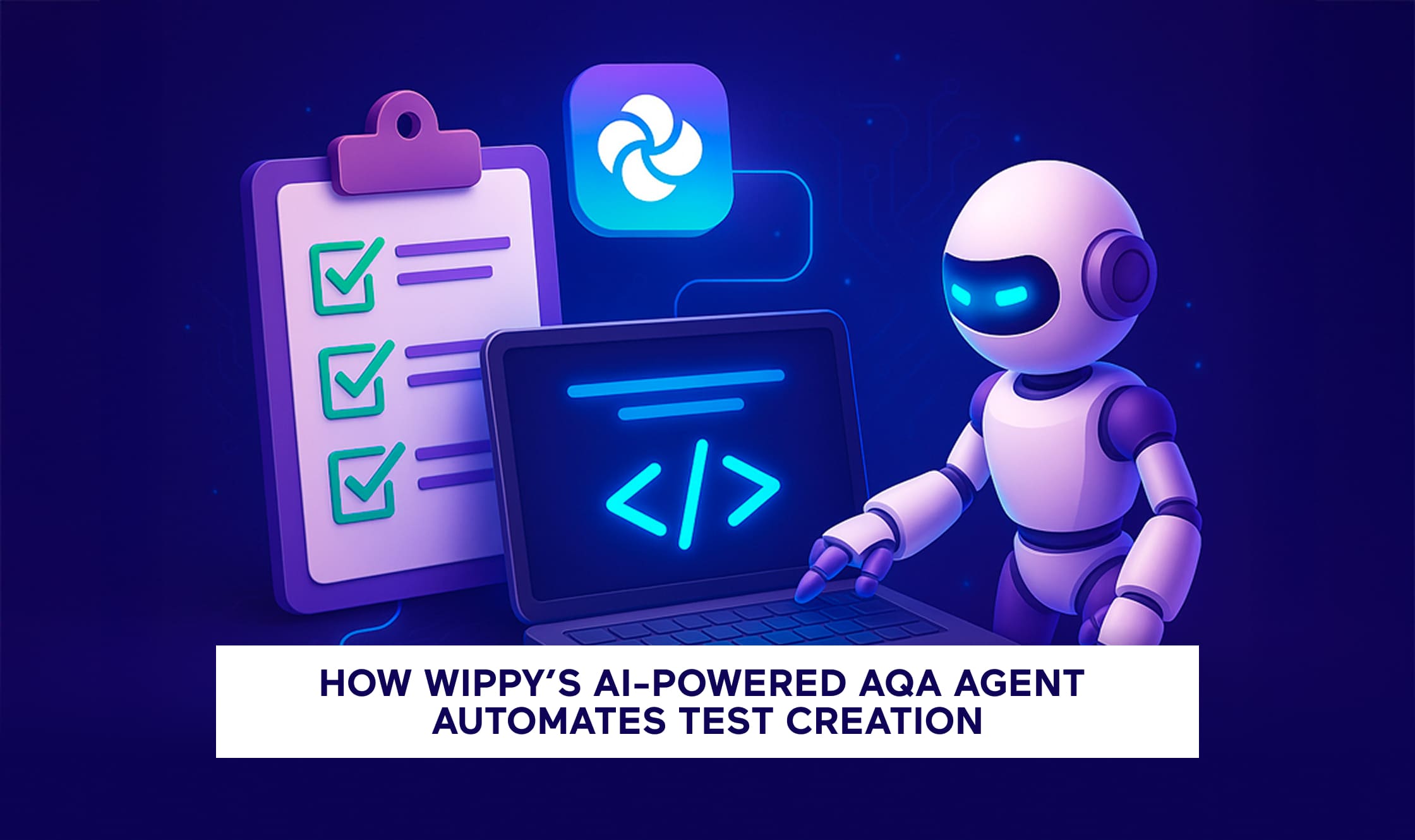
Reimagining QA: How Wippy’s AI-Powered AQA Agent Automates Testing from Spec to CI
Wippy’s AI-powered AQA Agent acts as a drop-in QA assistant that helps engineering and QA teams turn specs, tickets, and docs into runnable, framework-ready test scripts. From test scaffolding and flaky test handling to reporting and PR automation, this agent boosts velocity without needing to hire another engineer. What is the Wippy AQA Agent? The […]
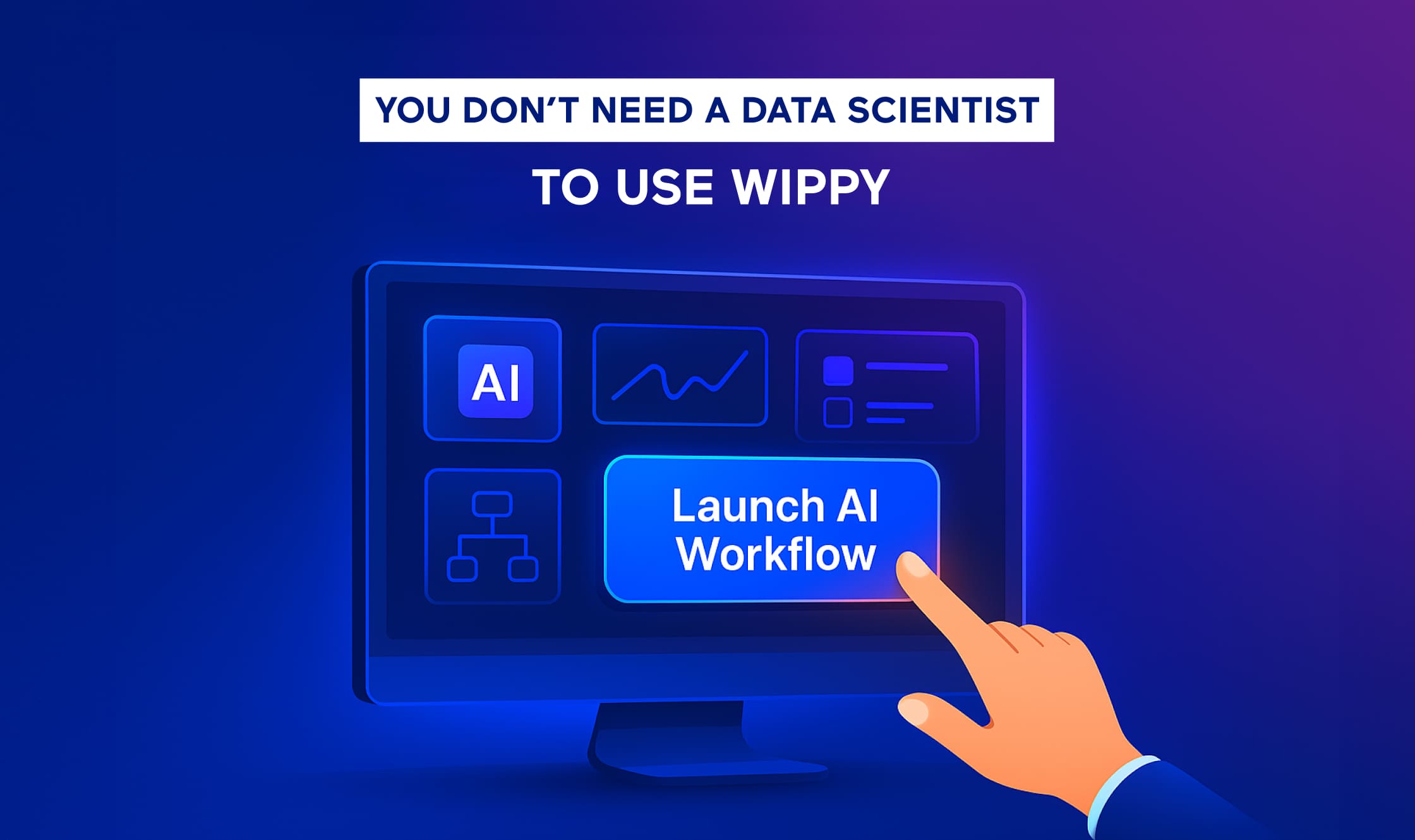
No Data Scientist Needed: Building AI Agents with Wippy for Business Teams
AI doesn’t have to be intimidating. With Wippy, non-technical teams can achieve massive benefits without needing a six-figure data scientist. This platform enables teams to build AI agents without coding, making AI accessible and practical for business operations. If you’re an entrepreneur or product manager exploring AI or agents, you’ve likely wondered, “Do we need […]
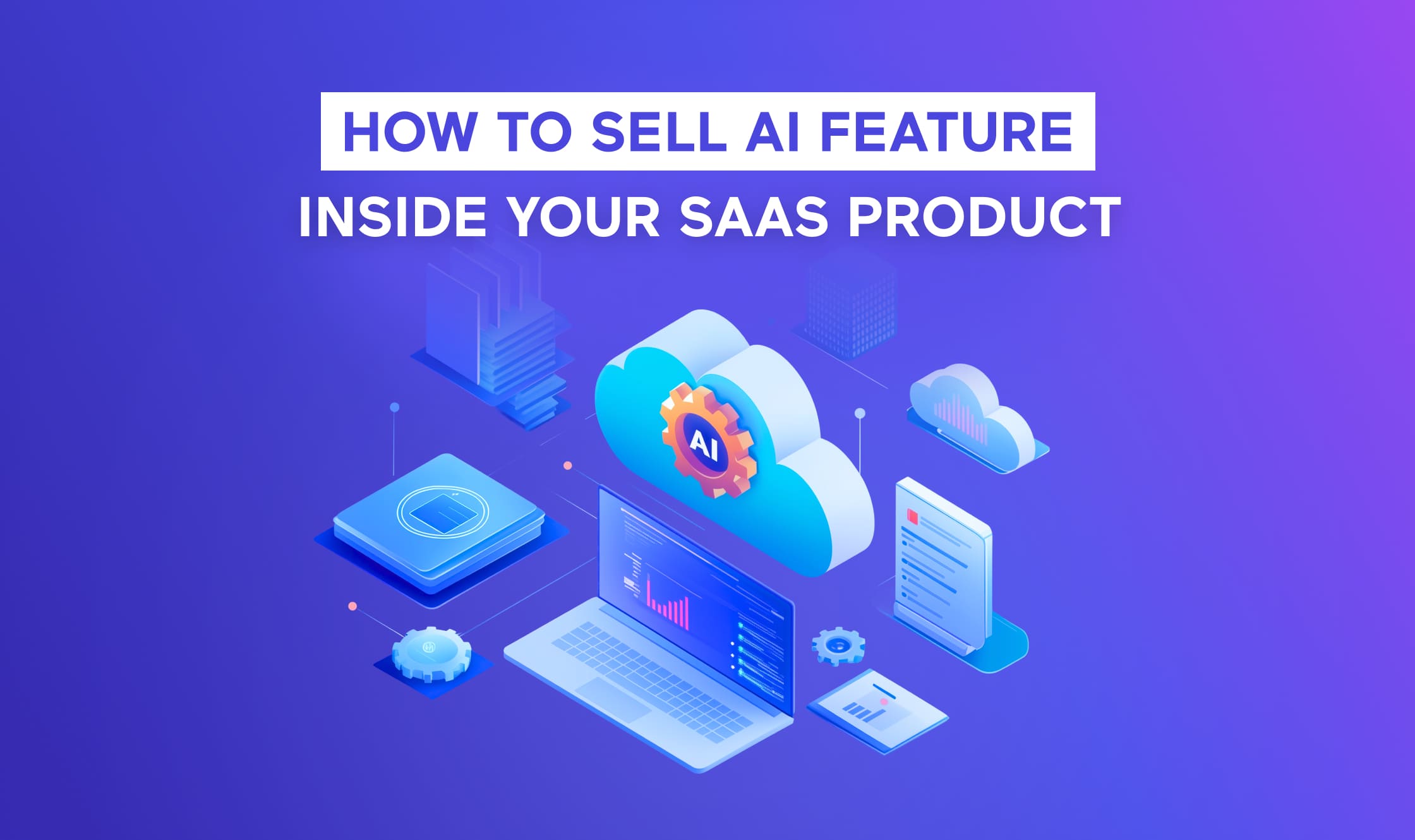
How Wippy-Powered AI Agents Open New Revenue for SaaS Businesses
There’s a growing trend among software-as-a-service providers: users now expect intelligent, responsive, and increasingly automated features. By leveraging the embedding AI into SaaS products and utilizing multi-agent frameworks, businesses can unlock new revenue streams and drive innovation. SaaS companies are witnessing a shift in user expectations. Customers are no longer just buying software; they want […]
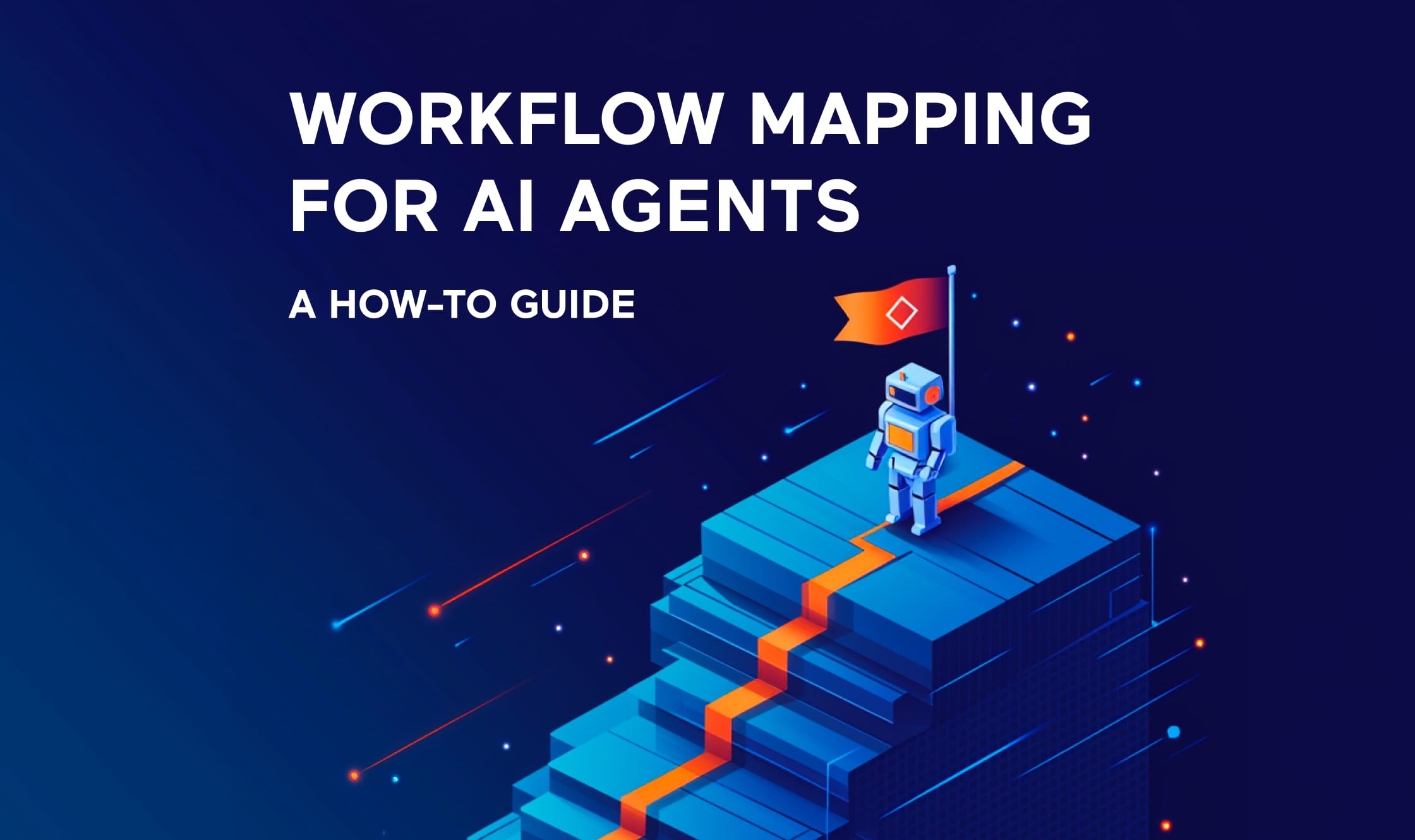
From Messy Processes to AI Agents: A Step-by-Step Guide
Many companies struggle with AI implementation not due to the technology itself, but because of unclear processes. By adopting agent workflow design best practices and leveraging AI consulting for businesses, you can transform complex tasks into well-structured AI agents. In our experience, the main reason companies encounter difficulties with AI isn’t the technology’s inadequacy. It’s […]
Subscribe to our newsletter
Recent posts
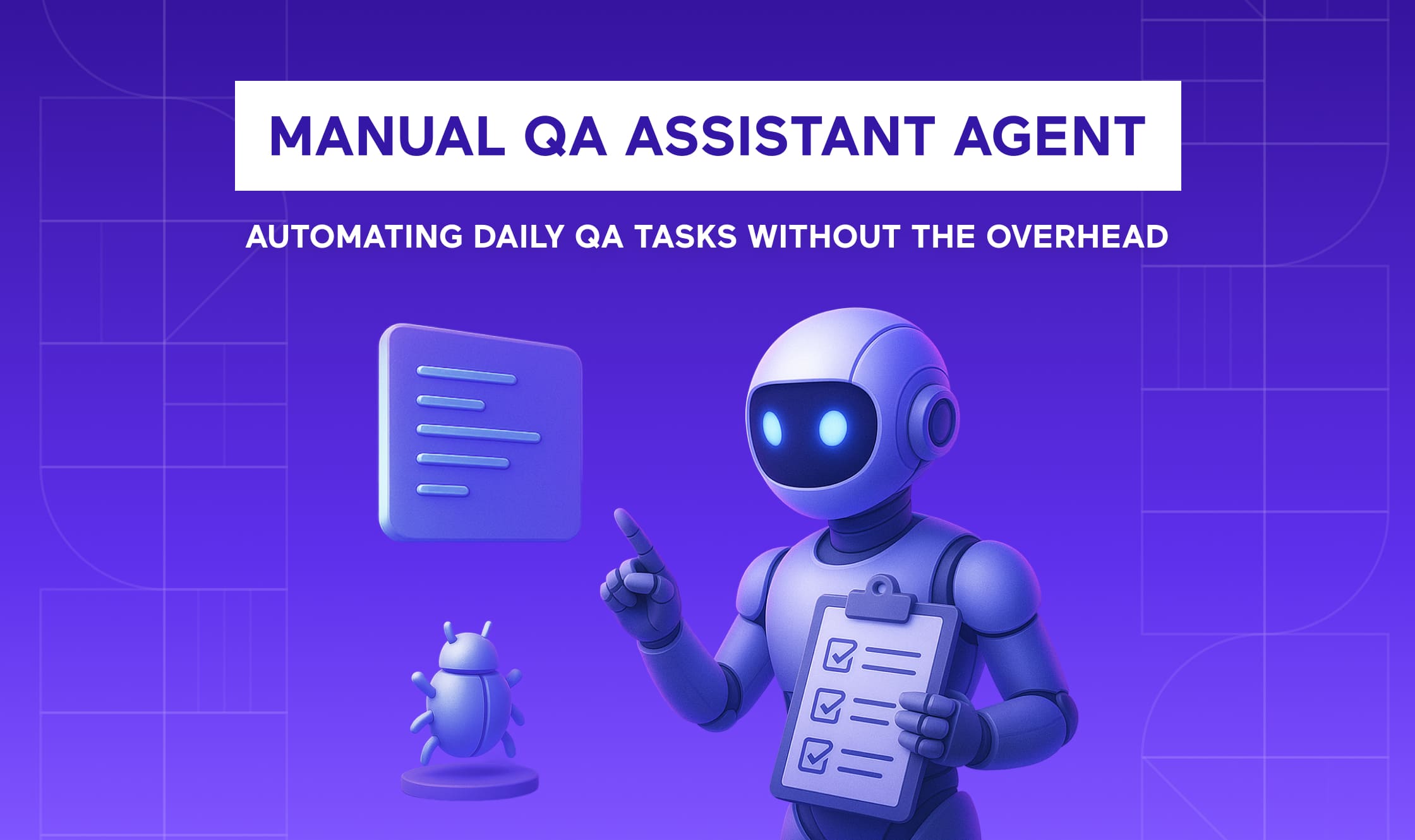
Meet the Manual QA Assistant Agent: Automating Daily QA Tasks Without the Overhead
Your manual QA team deserves better tools. Spiral Scout’s new AI-powered Manual QA Assistant Agent is built to help teams handle everyday grunt work – creating tickets, reading docs, extracting test data, scanning pages – so QAs can spend less time on busywork and more time ensuring product quality. What Does the Manual QA Assistant […]
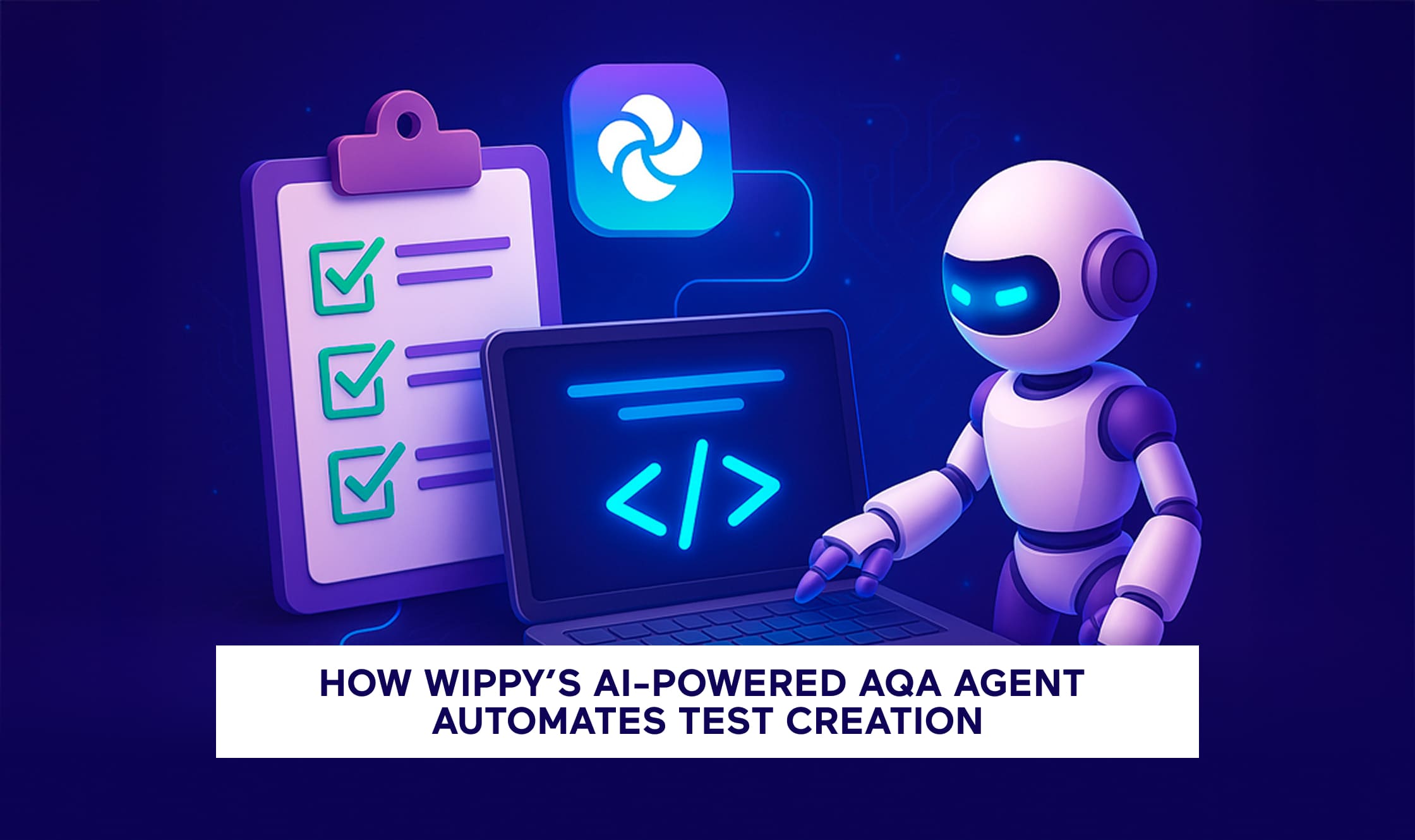
Reimagining QA: How Wippy’s AI-Powered AQA Agent Automates Testing from Spec to CI
Wippy’s AI-powered AQA Agent acts as a drop-in QA assistant that helps engineering and QA teams turn specs, tickets, and docs into runnable, framework-ready test scripts. From test scaffolding and flaky test handling to reporting and PR automation, this agent boosts velocity without needing to hire another engineer. What is the Wippy AQA Agent? The […]
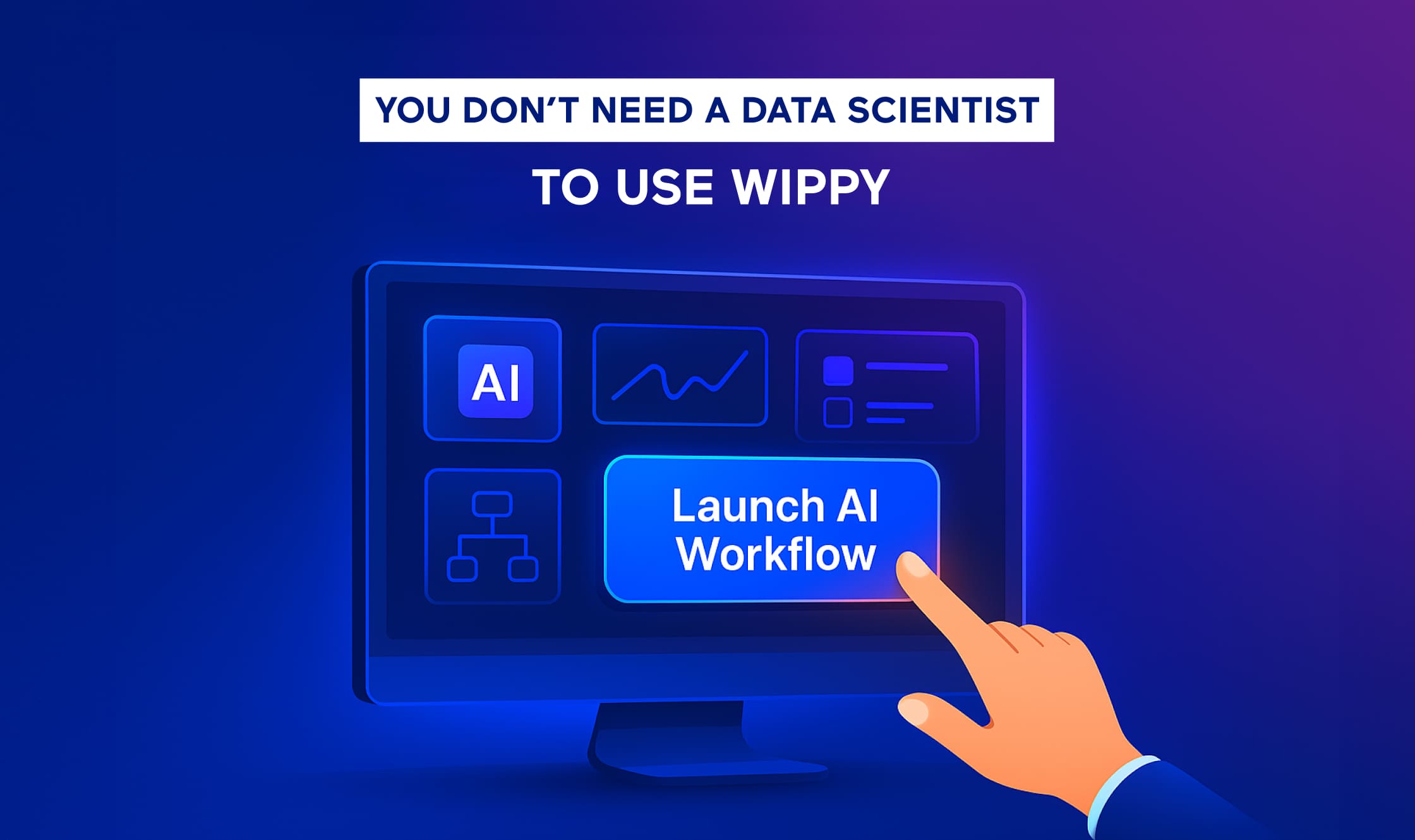
No Data Scientist Needed: Building AI Agents with Wippy for Business Teams
AI doesn’t have to be intimidating. With Wippy, non-technical teams can achieve massive benefits without needing a six-figure data scientist. This platform enables teams to build AI agents without coding, making AI accessible and practical for business operations. If you’re an entrepreneur or product manager exploring AI or agents, you’ve likely wondered, “Do we need […]
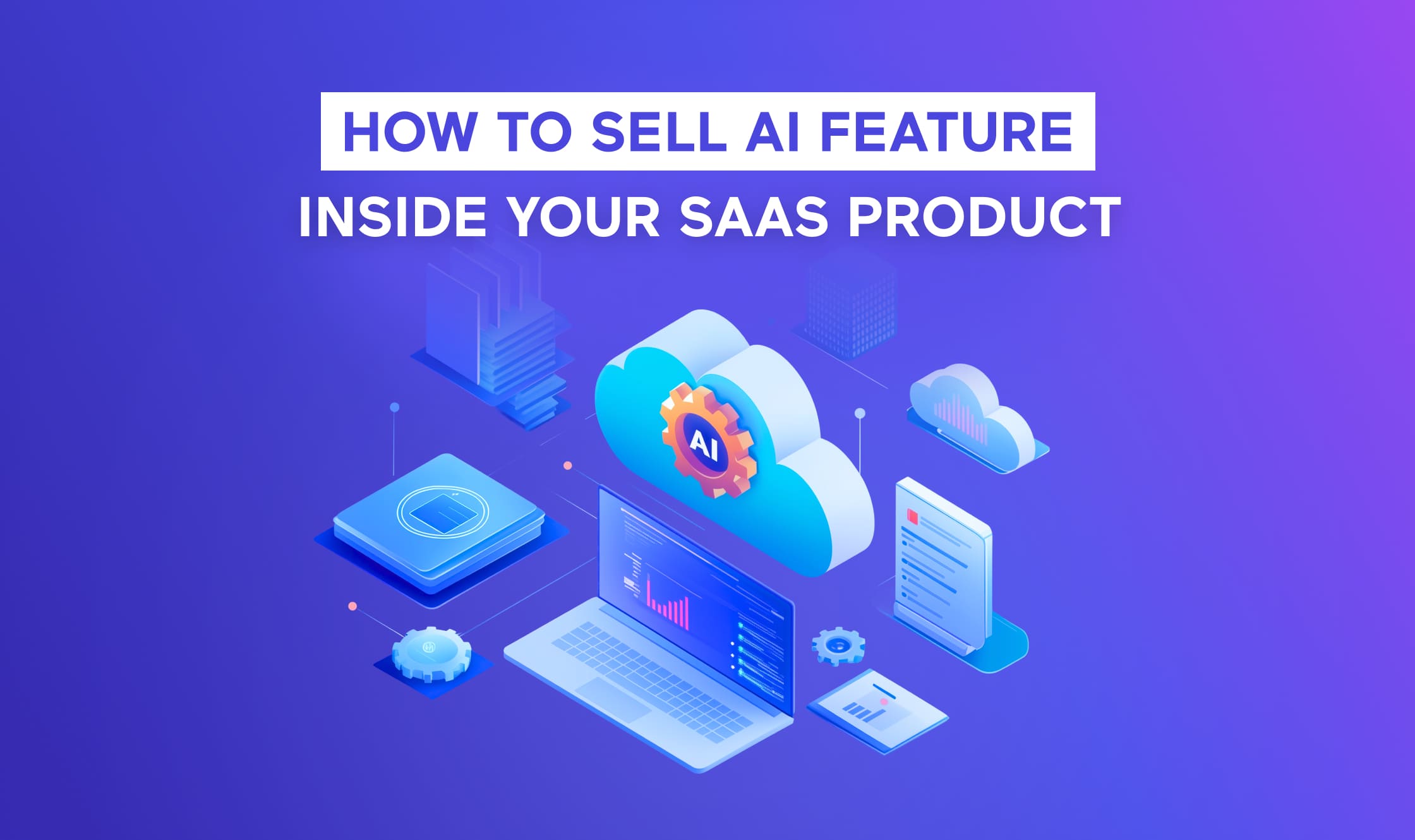
How Wippy-Powered AI Agents Open New Revenue for SaaS Businesses
There’s a growing trend among software-as-a-service providers: users now expect intelligent, responsive, and increasingly automated features. By leveraging the embedding AI into SaaS products and utilizing multi-agent frameworks, businesses can unlock new revenue streams and drive innovation. SaaS companies are witnessing a shift in user expectations. Customers are no longer just buying software; they want […]
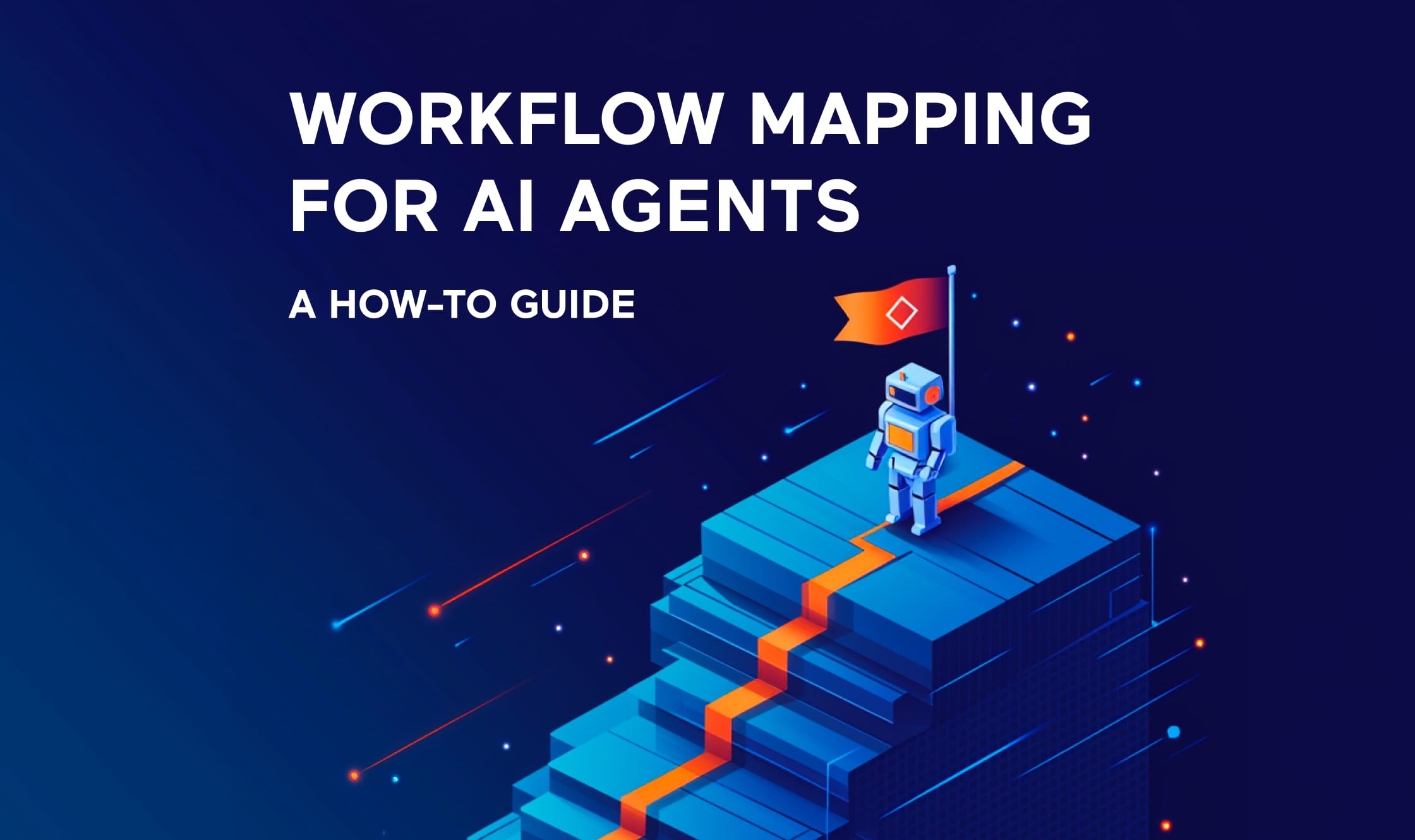
From Messy Processes to AI Agents: A Step-by-Step Guide
Many companies struggle with AI implementation not due to the technology itself, but because of unclear processes. By adopting agent workflow design best practices and leveraging AI consulting for businesses, you can transform complex tasks into well-structured AI agents. In our experience, the main reason companies encounter difficulties with AI isn’t the technology’s inadequacy. It’s […]
Turn your ideas into innovation.
Your ideas are meant to live beyond your mind. That’s what we do – we turn your ideas into innovation that can change the world. Let’s get started with a free discovery call.
Get Started
Get Started
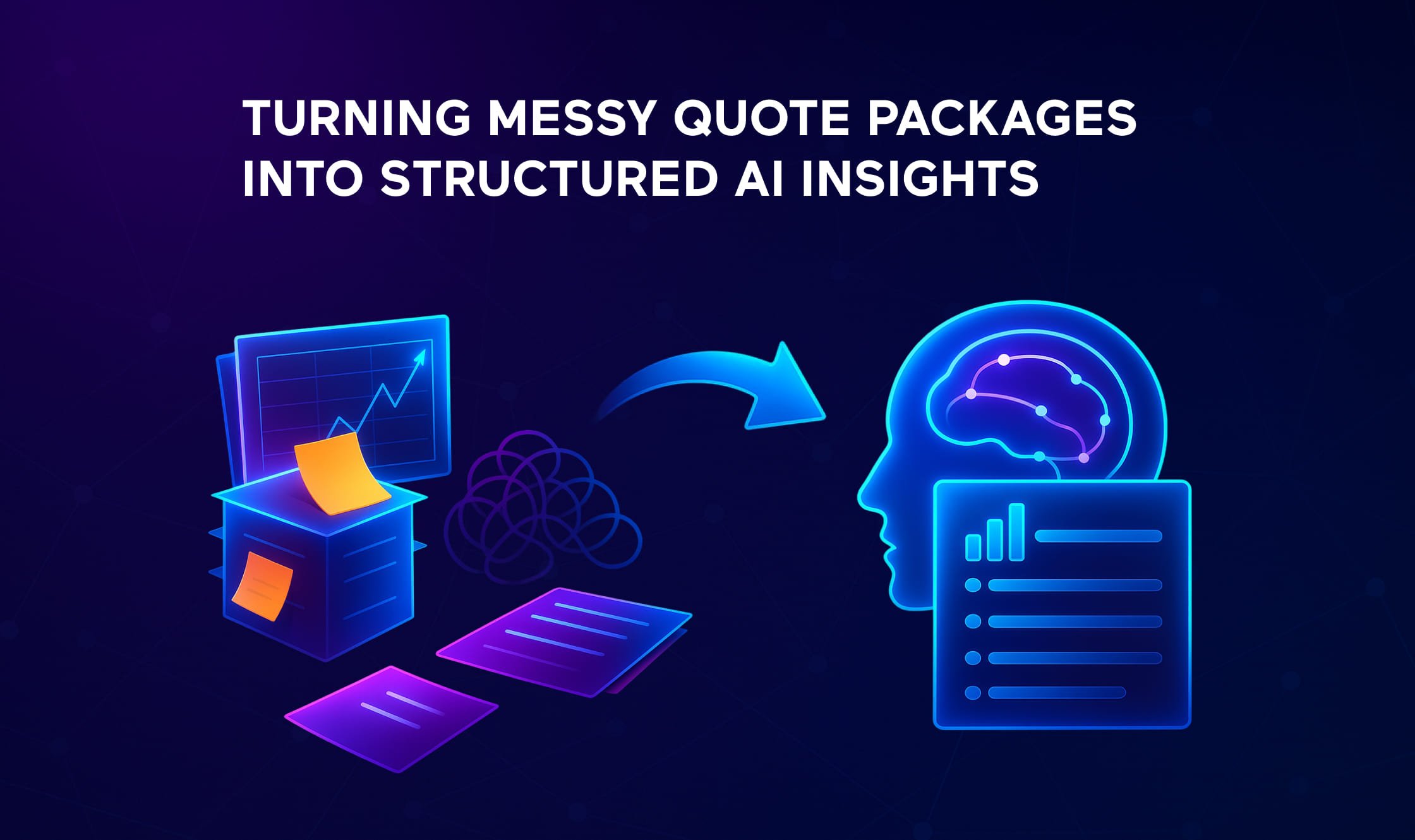
How AI Is Revolutionizing RFQ Automation in Manufacturing
AI is revolutionizing RFQ automation in manufacturing by enhancing speed, accuracy, and operational intelligence. Leveraging AI quoting automation for manufacturers and effective workflow mapping for RFQ AI agents can streamline processes and drive innovation. For manufacturers responding to complex RFQs from major clients, quoting isn’t just about pricing—it’s about speed and accuracy, as well as […]
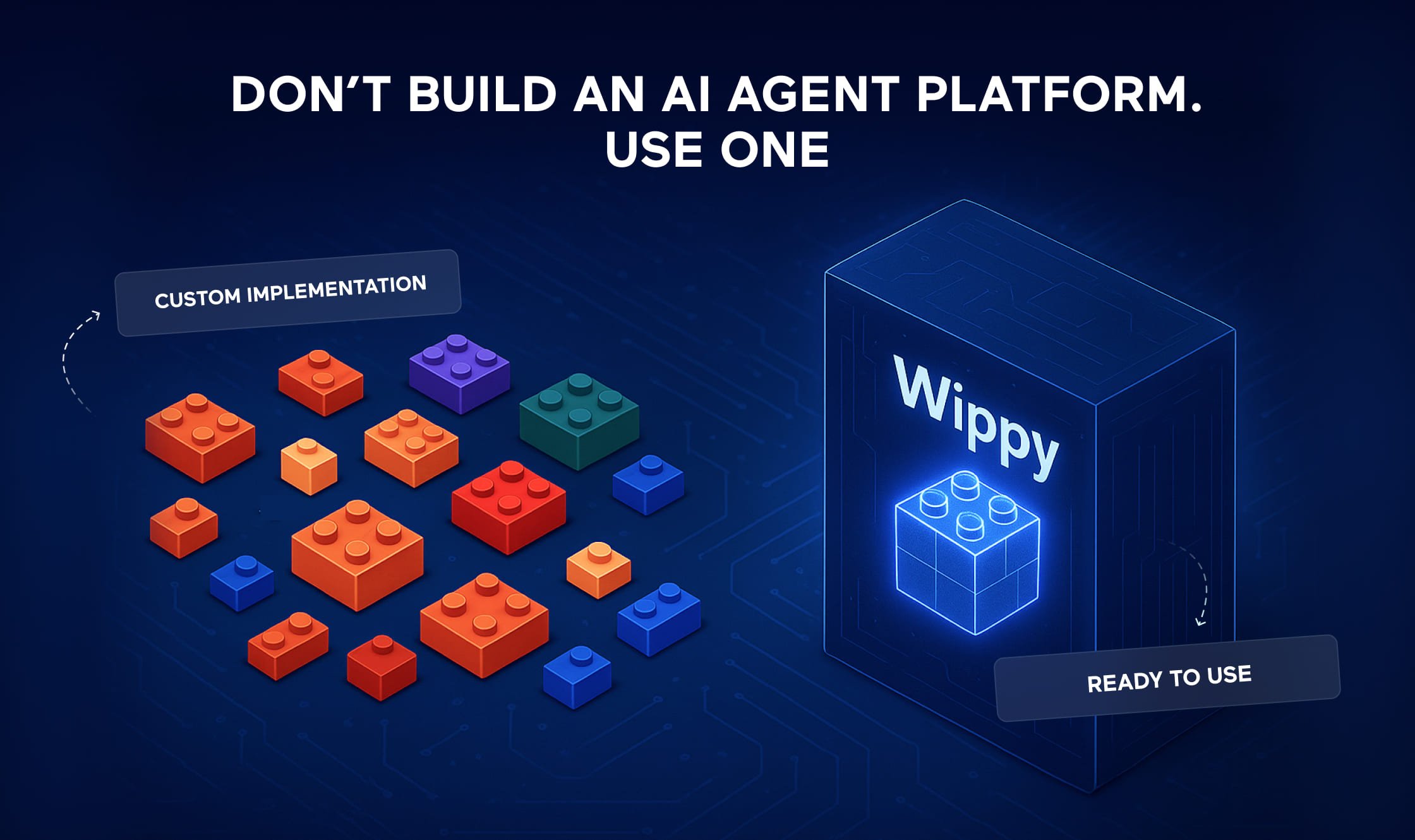
AI Platforms vs. DIY: Choosing the Smarter Path for Automation
AI platforms provide a smarter path to business automation compared to building from scratch. By leveraging purpose-built AI platforms and utilizing AI implementation services, businesses can achieve efficient and scalable solutions. You don’t need to be Palantir or build their software internally to get Palantir-level results. Building your own internal AI platform may seem appealing, […]
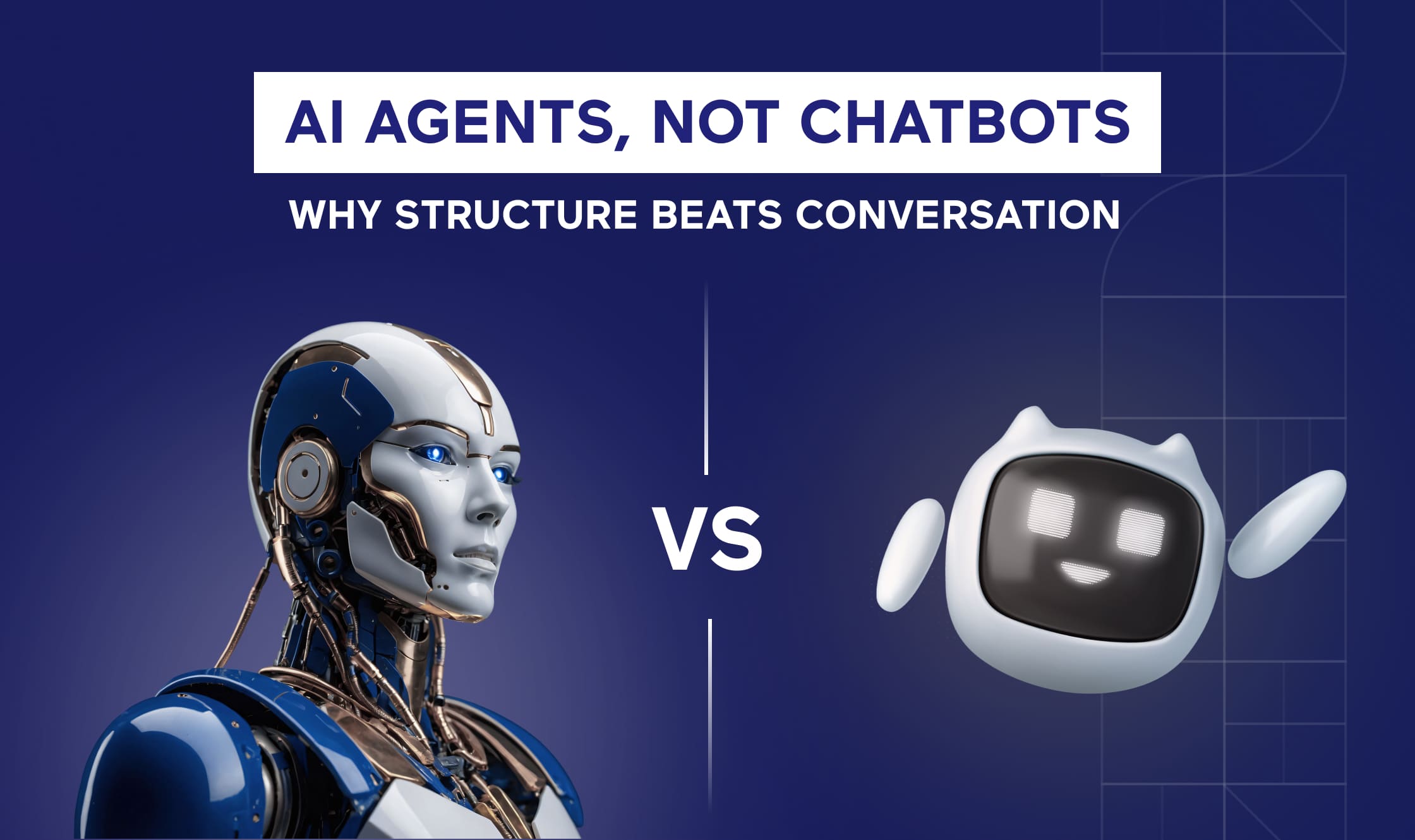
Structured AI Agents vs. Chatbots: How to Build Real Automation
Structured AI agents offer a superior approach to business automation compared to chatbots. By utilizing structured AI agents and enabling agent-powered quoting automation, businesses can achieve scalable, intelligent solutions. Most people’s first interaction with AI came through chatbots like ChatGPT—a reactive assistant that responds to queries in plain English. While useful for casual inquiries, chatbots […]