TIPS AND BEST PRACTICES
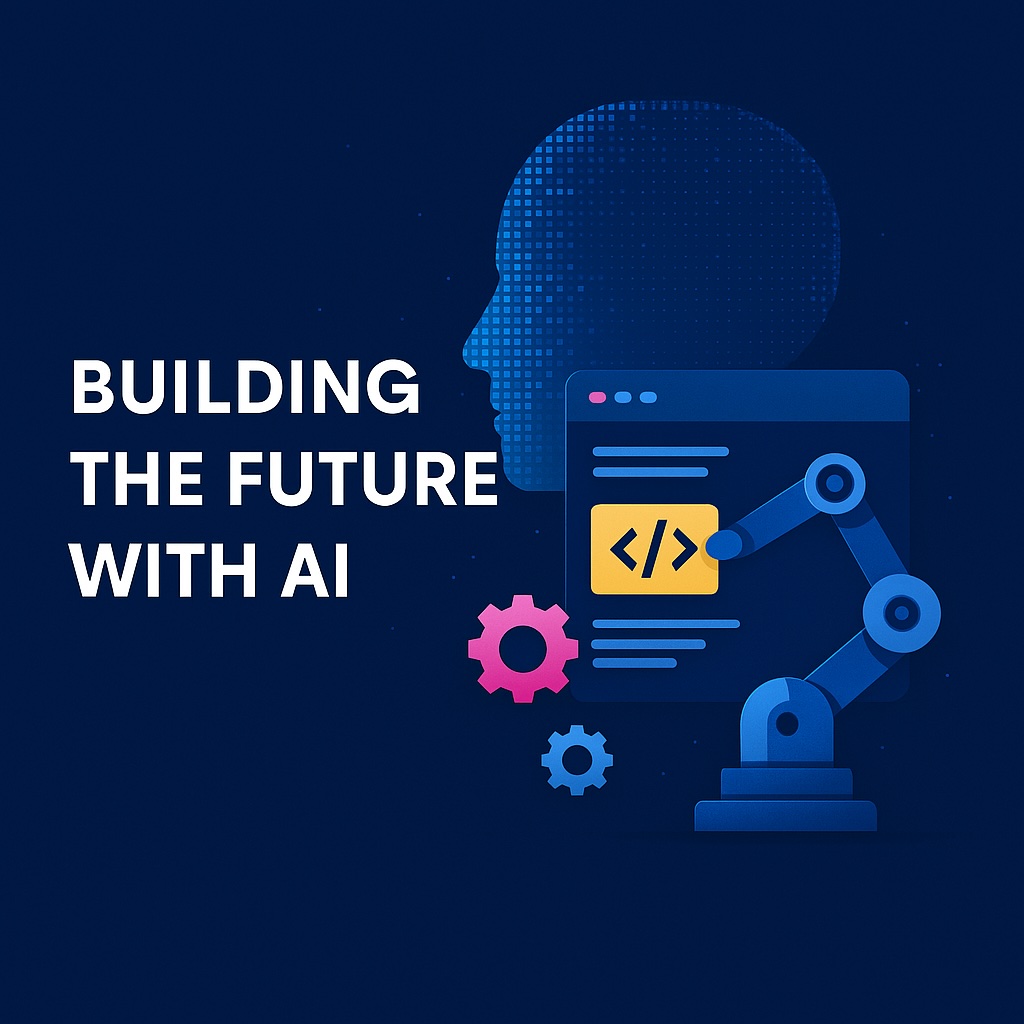
Building the Future with AI: How Spiral Scout Is Reimagining Self-Building Software
In a world where AI is reshaping the way software is created, deployed, and maintained, the question is no longer if we should integrate AI—but how. At Spiral Scout, that “how” is being answered with an ambitious, intelligent shift in direction: transforming Wippy from a powerful, agentic AI platform into the core of a new […]
Trending
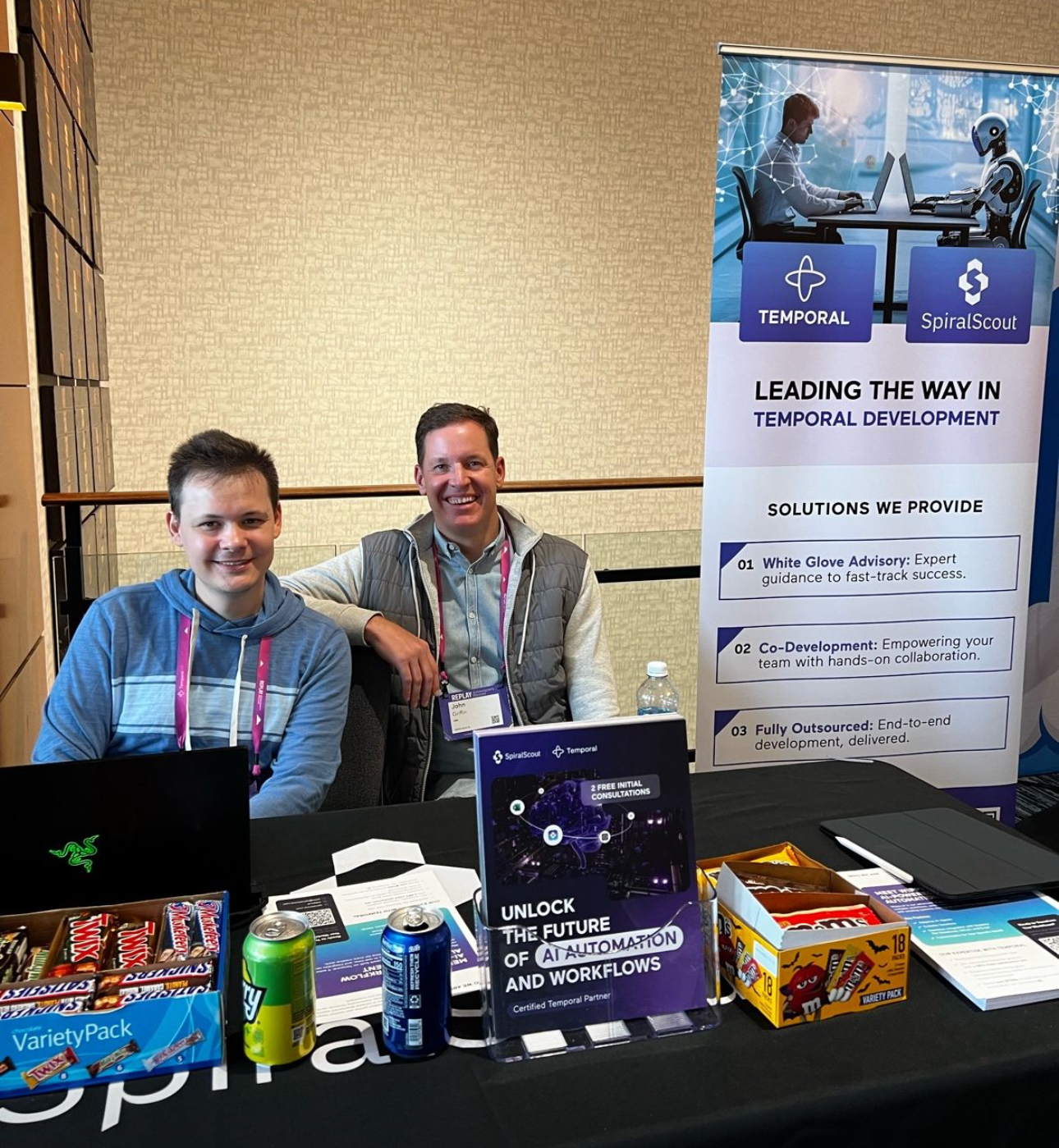
How AI is Transforming My Life and Work
The integration of AI into my daily routine has been nothing short of transformative. As the co-founder and investor in multiple business, it’s not just about saving time; it’s about augmenting my capabilities, solving problems faster, and finding creative solutions I wouldn’t have considered otherwise. Here’s how I’ve personally been leveraging AI tools to maximize […]
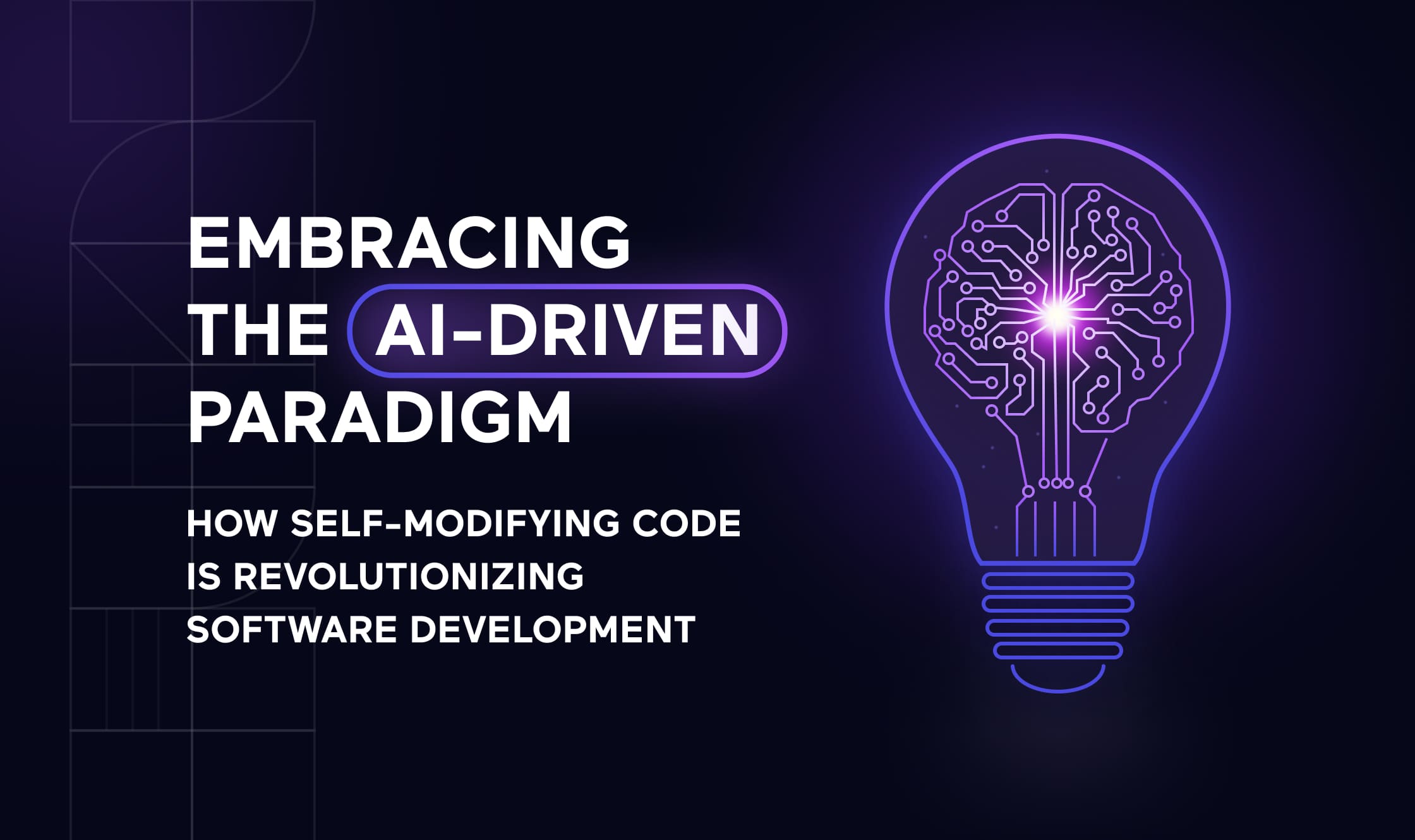
Embracing the AI-Driven Paradigm: How Self-Modifying Code is Revolutionizing Software Development
In the rapidly evolving software development landscape, a new paradigm is emerging—one that leverages artificial intelligence (AI) to fundamentally change how we write, maintain, and interact with code. This shift isn’t just about automating tasks or generating snippets; it’s about creating self modifying code for software that can understand and improve itself. Spiral Scout’s CTO […]
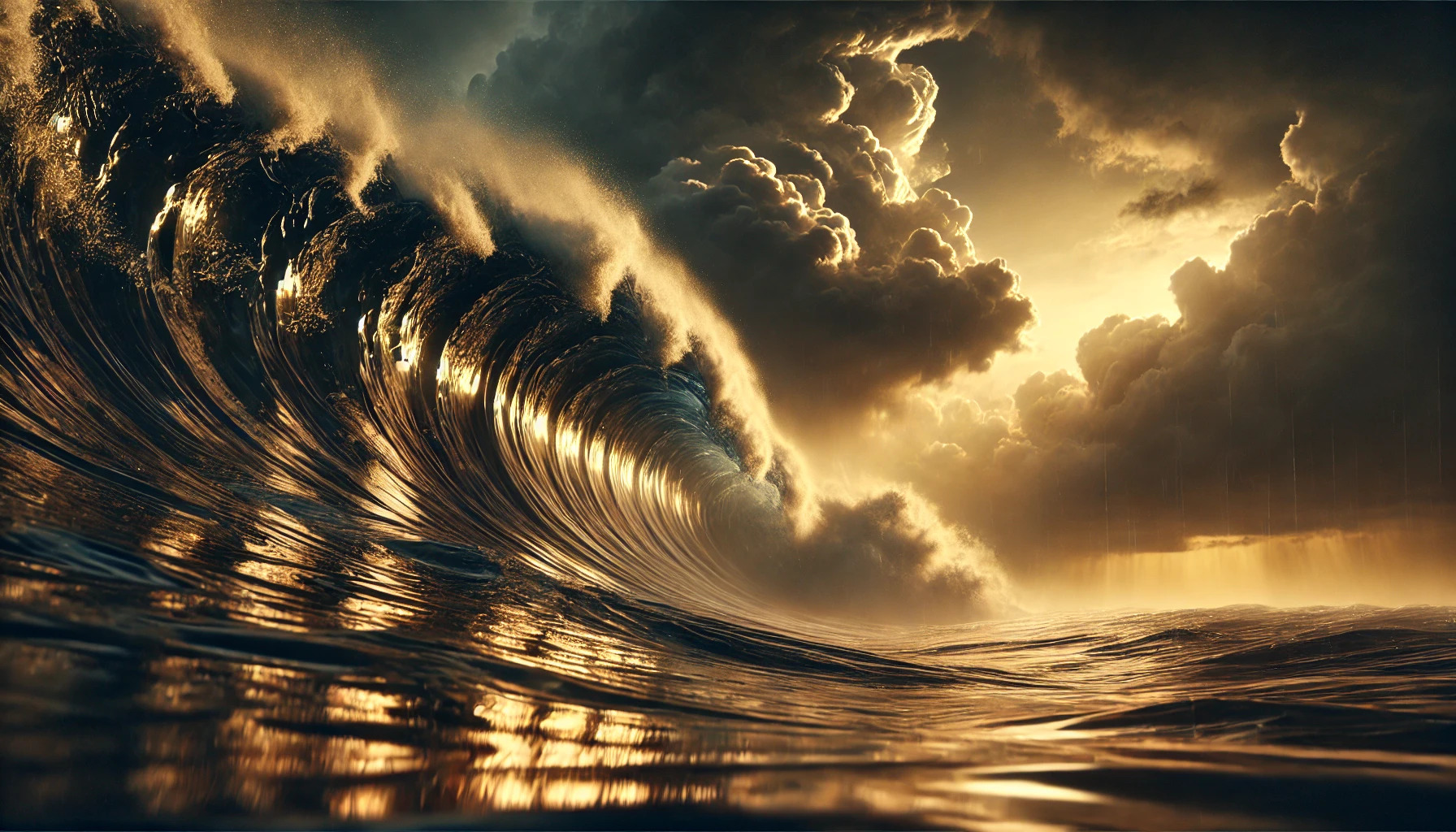
Wippy and the Future of AI-Powered Business Automation
The way businesses approach automation is changing fast. SaaS transformed software over the last 25 years, but now we’re seeing a shift toward AI-driven platforms that leverage digital employees or agents that blend software and services in ways SaaS never could. The rise of AI Agents Platforms (AIAPs) or Agents as a Service (AaaS) shows […]
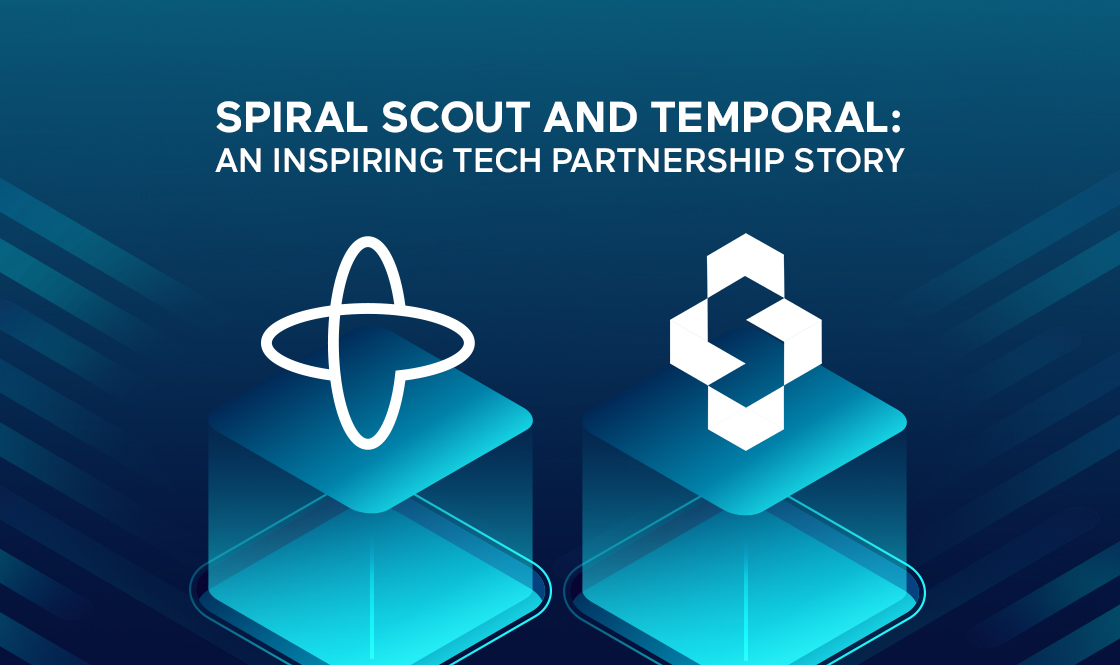
Spiral Scout and Temporal: An Inspiring Tech Partnership Story
Back in 2019, JD, Spiral Scout’s co-founder and CTO, was building his own workflow engine (like most engineers who have had similar thoughts that building and maintaining a workflow engine was a good idea). While he was starting to build this engine, he was researching different solutions on the market to get some ideas and […]
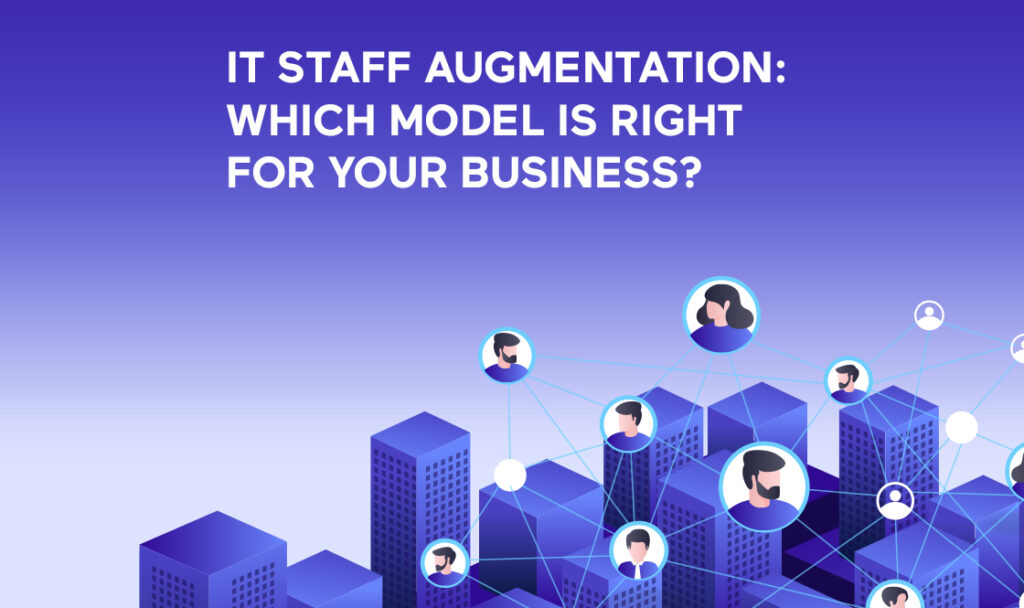
IT Staff Augmentation: Which Model is Right For Your Business?
Growing Your Business with IT Staff Augmentation Today, more and more organizations are using IT staff augmentation to access the right talent at the right time without the long-term financial implications of putting more employees on the payroll. Businesses need to implement technologies quickly and effectively in order to stay competitive. While it can be […]
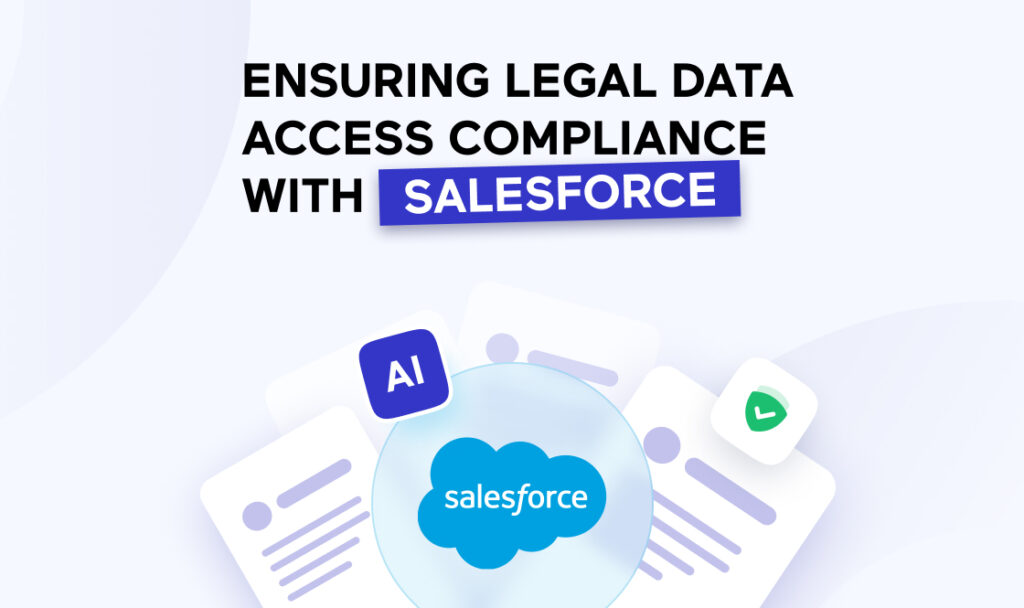
Master Legal Data Access Compliance in Salesforce
Ensuring Legal Data Access Compliance with Salesforce Dealing with complex data access laws is one of the most taxing responsibilities for legal teams today, regardless of your industry. With regulations constantly shifting across different regions and industries, you always run the risk of costly human errors – one overlooked detail can lead to hefty fines […]
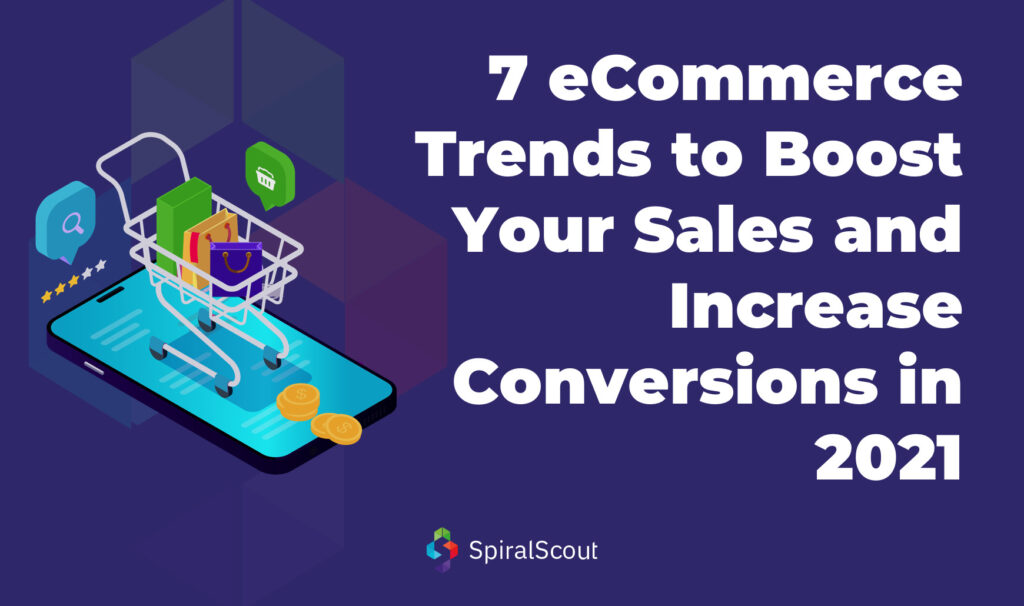
7 eCommerce Trends To Boost Your Sales and Increase Conversions
Top 2021 eCommerce Trends Deserving Your Attention in 2021 With the changes to our current digital age, eCommerce trends are popping up everywhere. Businesses that fail to learn about the changes and react intelligently to them risk falling behind their competition and losing out on business. COVID-19 dramatically increased the pace that legacy businesses needed […]
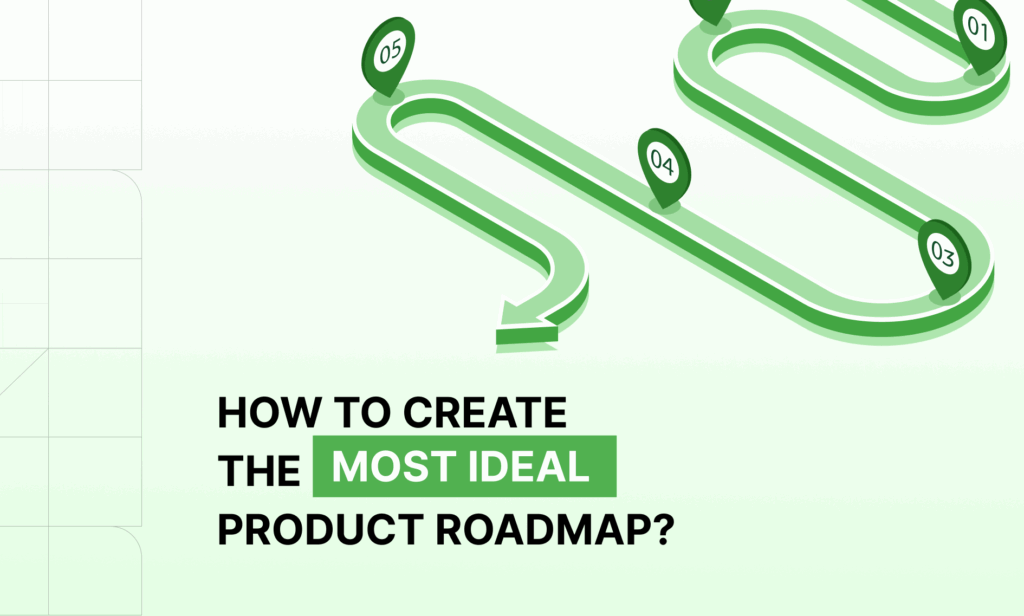
How to Create the Most Ideal Product Roadmap?
Having a great idea is only a part of what it takes to launch and scale a successful product. Product planning, clear communication, and executing on the plan among many stakeholders are equally as important. For a product manager, it’s essential to involve the whole team in brainstorming ideas, project planning, and organization while still […]
Editor’s pick
AI Expertise
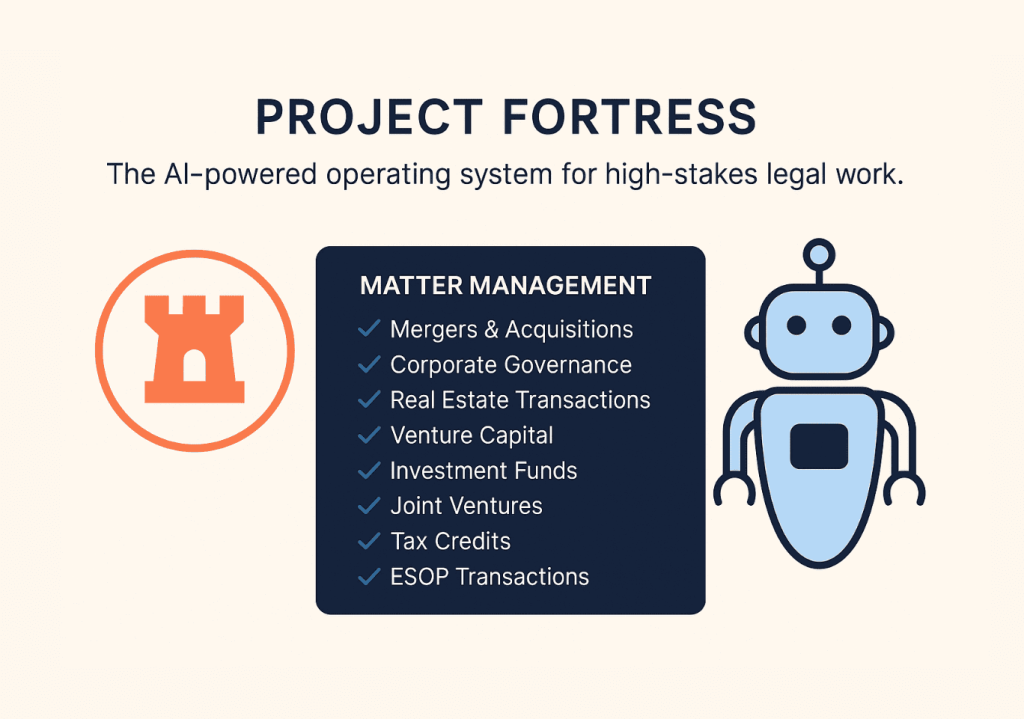
Project Fortress: How AI Agents Are Redefining Legal Workflows from the Ground Up
Law firms—especially those focused on transactional matters—are drowning in complexity. From coordinating due diligence and drafting schedules to tracking deal terms and managing communication across dozens of stakeholders, the operational overhead is immense. That’s exactly why Project Fortress exists. Built on top of the Wippy AI framework, Fortress isn’t just another legal tech tool—it’s a […]
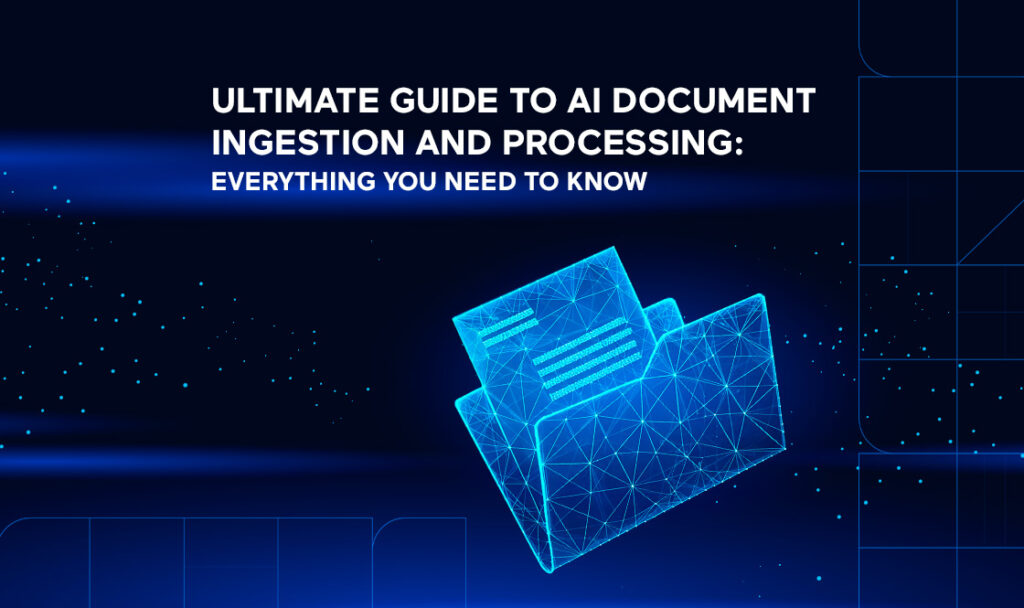
Ultimate Guide to AI Document Ingestion and Processing: Everything You Need to Know
Data batches filled with contracts, customer feedback, invoices, multimedia files—all of that will take human hours or even days to process. Luckily, though, recent years have seen the development of advanced AI solutions that can do the same job with almost the same accuracy within seconds.That’s why it’s not surprising to witness the Intelligent Document […]
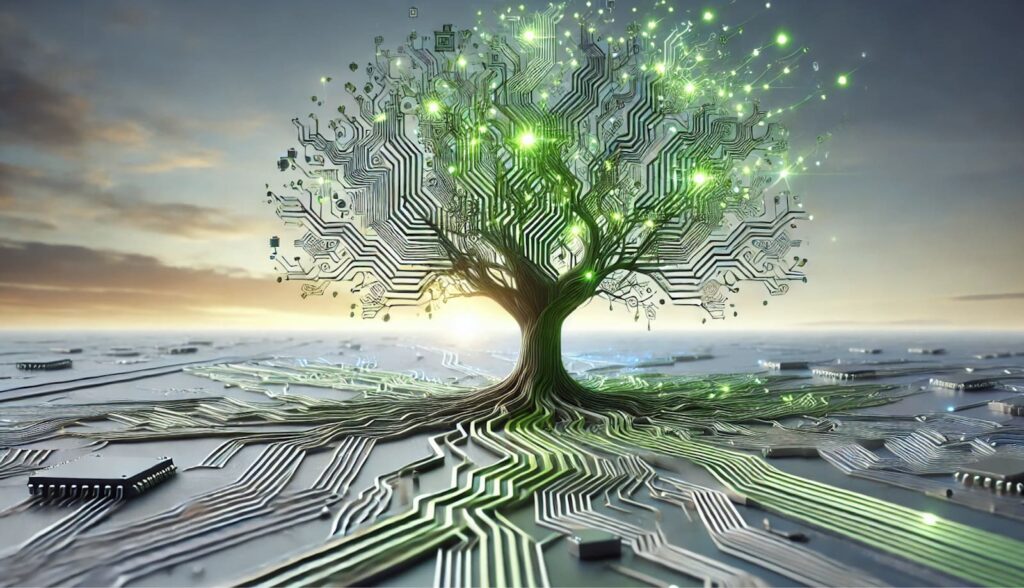
A New Phase in AI: Self-Improving Systems and Rapid Knowledge Acquisition
The AI community often spotlights generative models, chatbots, and application-centric agents, but one key innovation remains overlooked: rapid knowledge acquisition through self-modifying code. Recent conversations within Spiral Scout and our extended network highlight the growing importance of AI systems that can learn on the fly, change their own codebase, and adapt to new domains almost […]
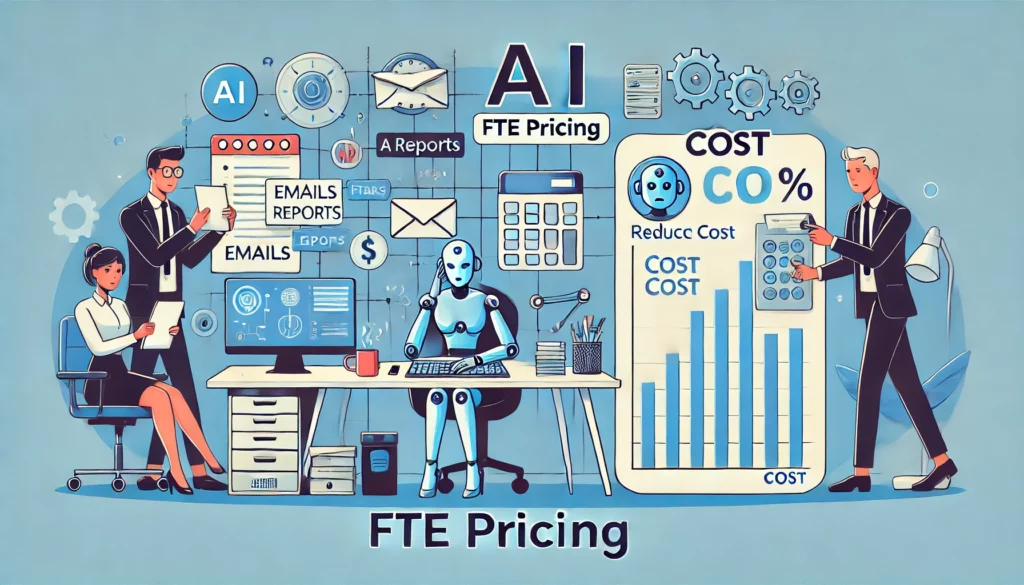
Transforming AI Monetization: The Rise of the “AI FTE” Pricing Model
In the evolving world of AI monetization and how best to use AI in practical ways at your company, a fascinating trend is emerging: services businesses or AI software companies are charging per AI Full-Time Equivalent (FTE) hired. This model borrows heavily from traditional hiring frameworks by mapping the output of human employees and translating […]
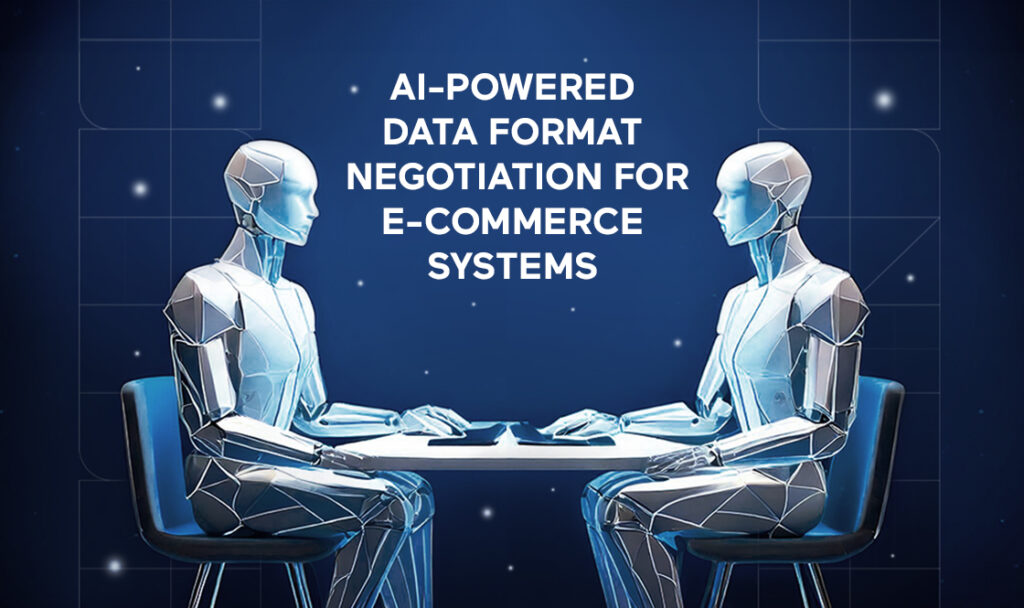
AI-Powered Data Format Negotiation for E-Commerce Systems
AI-Powered Strategies for Data Format Negotiation in Large-Scale E-Commerce Systems A typical e-commerce platform handles thousands of transactions daily, connecting to multiple payment gateways, shipping services, and inventory systems. In a system like this even a tiny discrepancy in the product data feed format, like a date written differently in one system, can cause a […]
Subscribe to our newsletter
Recent posts
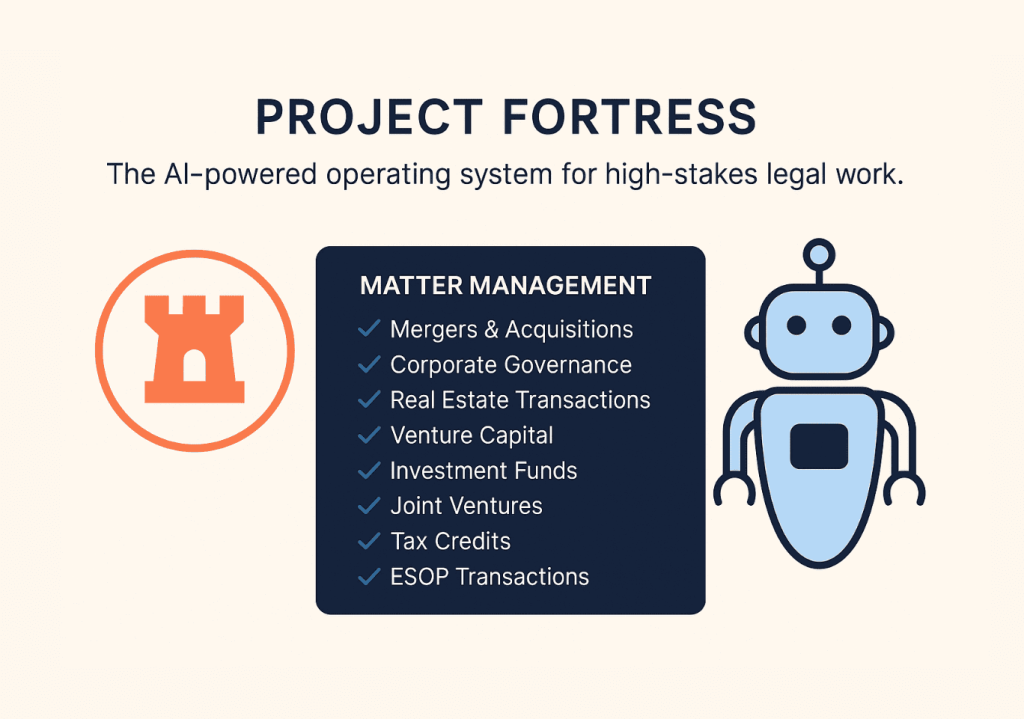
Project Fortress: How AI Agents Are Redefining Legal Workflows from the Ground Up
Law firms—especially those focused on transactional matters—are drowning in complexity. From coordinating due diligence and drafting schedules to tracking deal terms and managing communication across dozens of stakeholders, the operational overhead is immense. That’s exactly why Project Fortress exists. Built on top of the Wippy AI framework, Fortress isn’t just another legal tech tool—it’s a […]
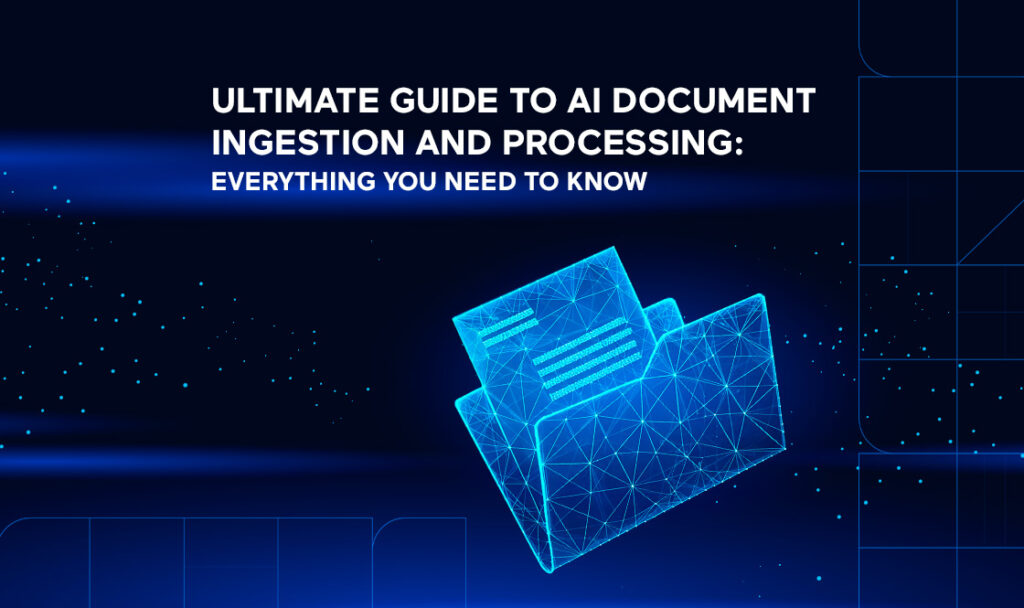
Ultimate Guide to AI Document Ingestion and Processing: Everything You Need to Know
Data batches filled with contracts, customer feedback, invoices, multimedia files—all of that will take human hours or even days to process. Luckily, though, recent years have seen the development of advanced AI solutions that can do the same job with almost the same accuracy within seconds.That’s why it’s not surprising to witness the Intelligent Document […]
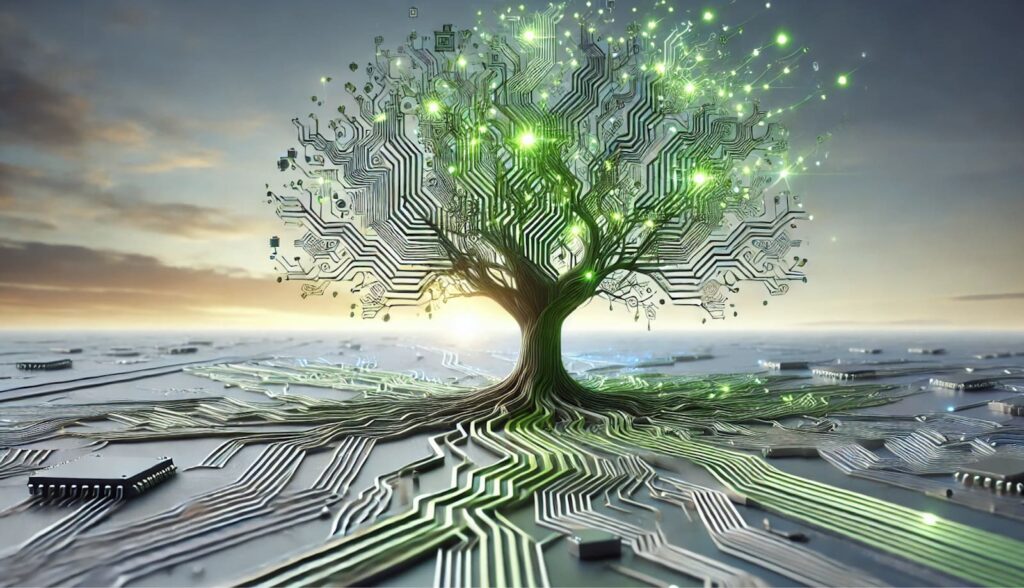
A New Phase in AI: Self-Improving Systems and Rapid Knowledge Acquisition
The AI community often spotlights generative models, chatbots, and application-centric agents, but one key innovation remains overlooked: rapid knowledge acquisition through self-modifying code. Recent conversations within Spiral Scout and our extended network highlight the growing importance of AI systems that can learn on the fly, change their own codebase, and adapt to new domains almost […]
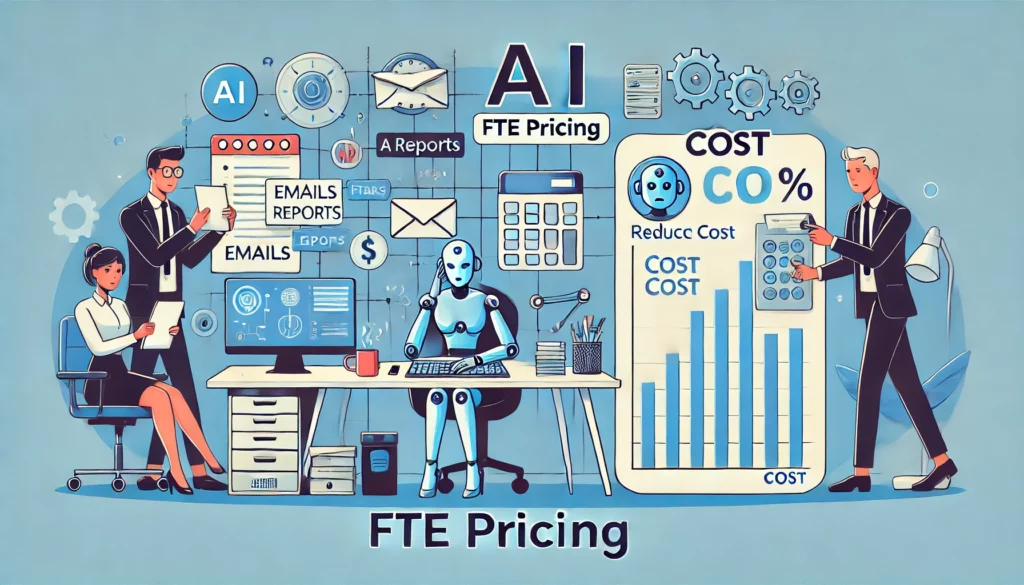
Transforming AI Monetization: The Rise of the “AI FTE” Pricing Model
In the evolving world of AI monetization and how best to use AI in practical ways at your company, a fascinating trend is emerging: services businesses or AI software companies are charging per AI Full-Time Equivalent (FTE) hired. This model borrows heavily from traditional hiring frameworks by mapping the output of human employees and translating […]
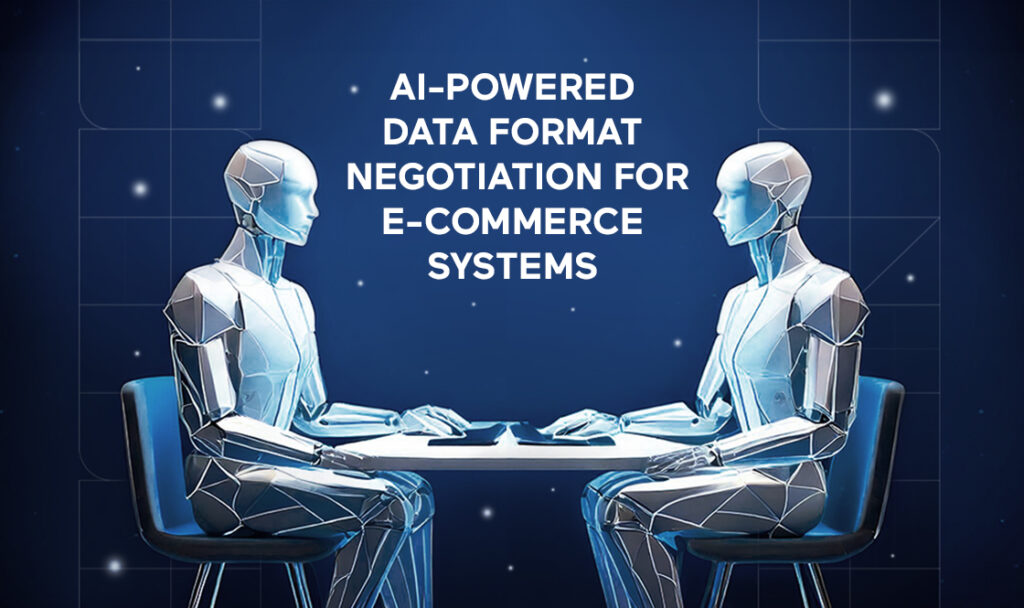
AI-Powered Data Format Negotiation for E-Commerce Systems
AI-Powered Strategies for Data Format Negotiation in Large-Scale E-Commerce Systems A typical e-commerce platform handles thousands of transactions daily, connecting to multiple payment gateways, shipping services, and inventory systems. In a system like this even a tiny discrepancy in the product data feed format, like a date written differently in one system, can cause a […]
Turn your ideas into innovation.
Your ideas are meant to live beyond your mind. That’s what we do – we turn your ideas into innovation that can change the world. Let’s get started with a free discovery call.
Get Started
Get Started
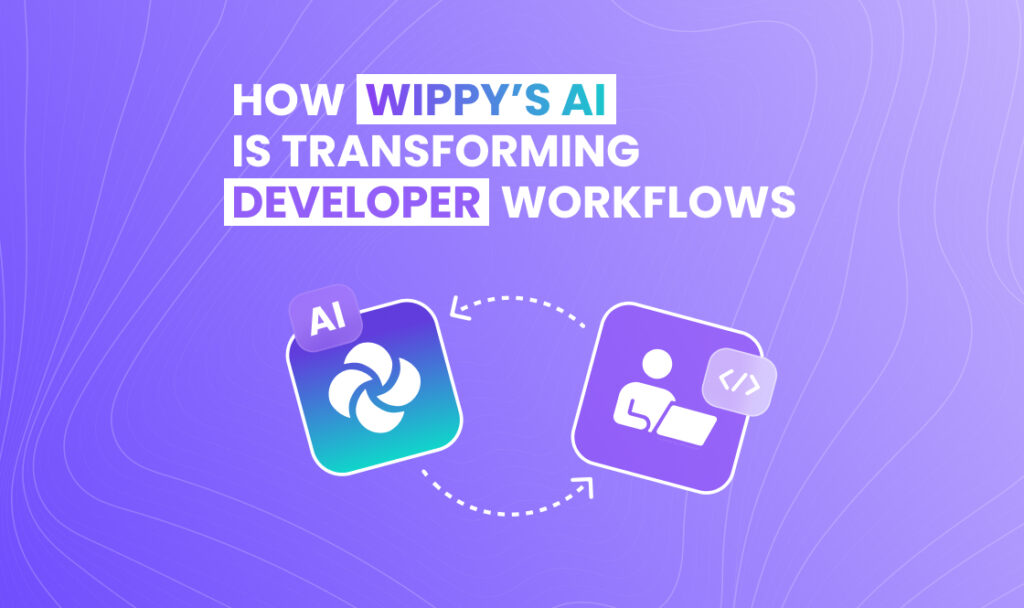
How Wippy is Transforming the Role of Developers in the Age of AI
Whether AI will eliminate professional services jobs and more specifically engineering jobs is a topic of much debate. Among the software developers themselves, the consensus is that their roles are not under imminent threat, but they are going to have to upskill their abilities and knowledge around how to use AI. I found it very […]
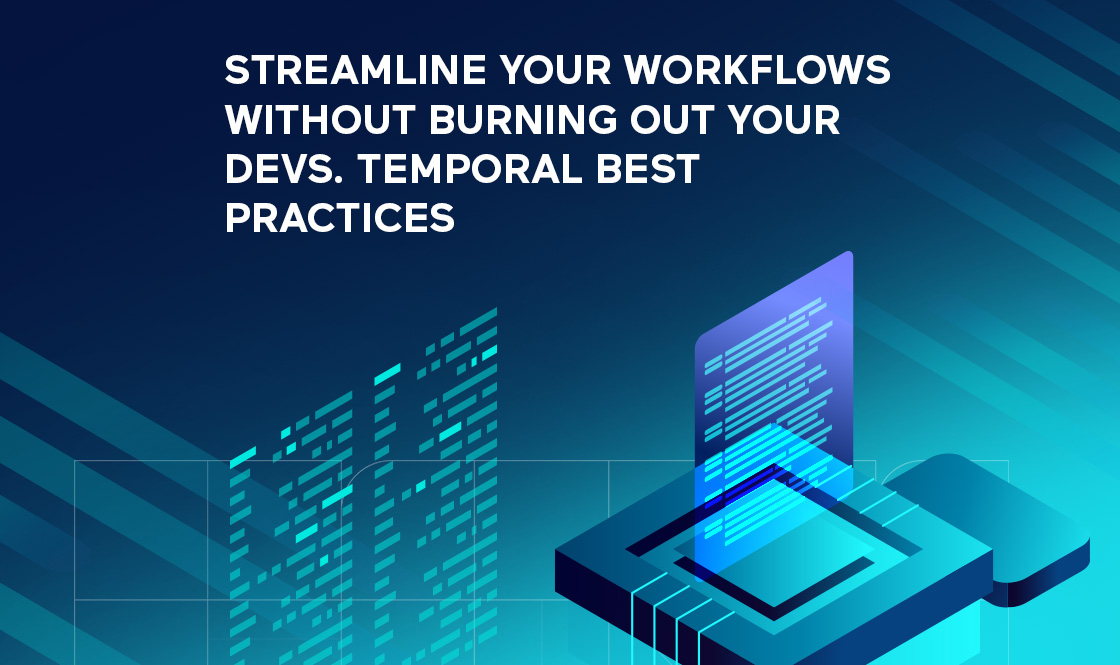
How to streamline your workflows without burning out your devs: a guide to Temporal.io
When it comes to workflows, you’ve probably automated some—or a lot—of them. You may have even built your own workflow engine. (Or considered doing it.) Automating workflows is a game-changer for businesses looking to build world-class software, scale, and spend more time on their core products instead of maintaining multiple custom workflows. But as those […]
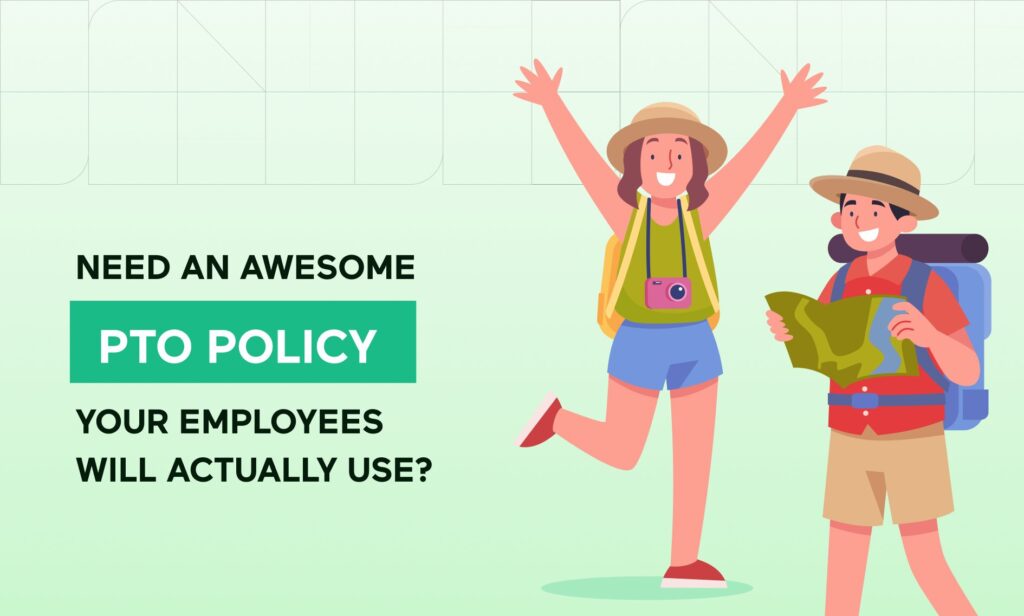
Need an Awesome PTO Policy Your Employees Will Actually Use?
Did you know that the U.S. does not have a statutory minimum annual leave requirement? Unlike many European countries that mandate companies offer their employees 20 to even 30 days of paid vacation each year, the U.S. has never instituted a similar policy. This diligent work ethic that has laid the foundation for innovation and […]